Automation Testing Of Application Using MongoDB
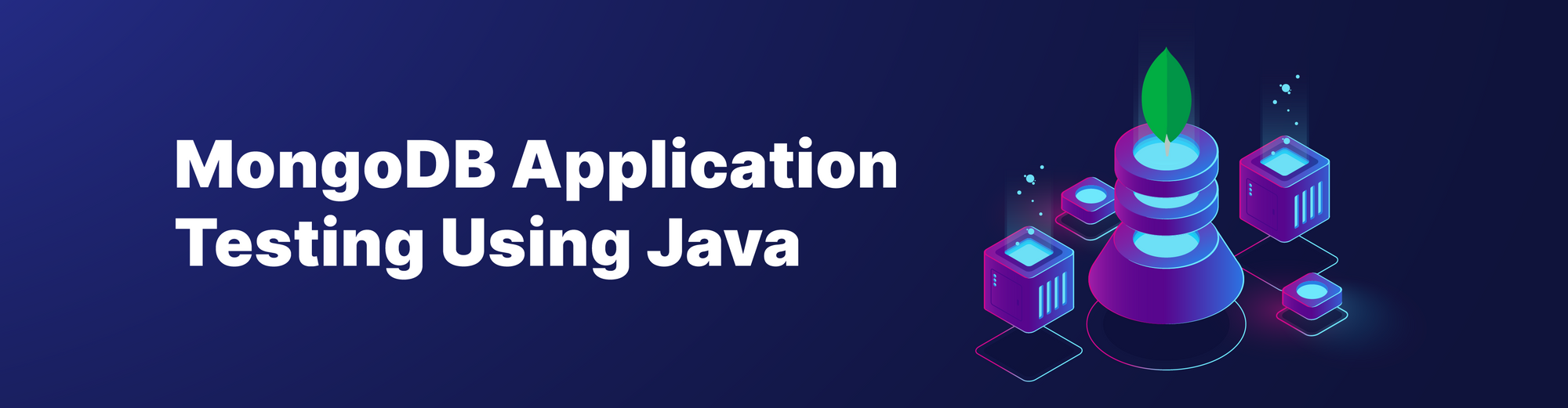
In the modern software development landscape, automation testing is a crucial practice that ensures the reliability and efficiency of applications. When working with modern databases like MongoDB, integrating automation testing can help in maintaining data integrity and performance. This blog will guide you through the process of setting up and performing automation testing for an application that uses MongoDB, leveraging the power of Java.
Table Of Contents
- Introduction to MongoDB Applications
- Why Automation Testing for MongoDB Application?
- Java Libraries Used for MongoDB Testing
- MongoDB Environment setUp and Connection
- Step-by-Step Guide to Test MongoDB Database
Introduction to MongoDB Applications
MongoDB is a leading NoSQL database known for its flexibility, scalability, and ability to handle large volumes of unstructured data. Unlike traditional relational databases, MongoDB stores data in JSON-like documents, making it highly adaptable to changing data schemas. This document-oriented storage approach allows developers to build and iterate on applications quickly. MongoDB is widely used in modern web, mobile, and cloud applications where speed and scalability are crucial. It supports complex querying and indexing, making it suitable for a wide range of applications, from content management systems to real-time analytics. With built-in replication and horizontal scaling, MongoDB ensures high availability and performance across distributed systems.
Why Automation Testing for MongoDB Application?
- Data Validation: Automation testing ensures that the data stored in MongoDB is accurate and consistent across various operations.
- Performance Monitoring: Regular automated tests can help in monitoring the performance of MongoDB queries and operations.
- Regression Testing: Automation helps in quickly identifying issues introduced by new changes, ensuring that the existing functionality is not broken.
- Continuous Integration: Automated tests can be integrated into CI/CD pipelines, ensuring that all code changes are tested before deployment.
Java Libraries Used for MongoDB Testing
Using java libraries for MongoDB automation offers a range of benefits that leverage Java's robust ecosystem, performance, and versatility. Here are several key reasons that Java is an excellent choice for automating MongoDB operations:
- Strong Typing and Compile-Time Checking: Java's strong typing system helps catch errors at compile-time, reducing the likelihood of runtime errors. This is especially useful in database operations where data integrity and type correctness are critical.
- Rich Ecosystem and Libraries: Java boasts a rich ecosystem of libraries and frameworks that simplify the development process. For MongoDB automation, Libraries like the MongoDB Java Driver and Spring Data MongoDB simplify automation.
- Integration with Build Tools: Java integrates well with build automation tools like Maven and Gradle. These tools manage dependencies, build processes, and continuous integration pipelines, making it easier to automate MongoDB operations as part of a larger automated workflow.
- Mature Testing Frameworks: Java has a mature ecosystem of testing frameworks such as JUnit and TestNG. These frameworks support the creation of comprehensive test suites for MongoDB operations, ensuring that database interactions are reliable and correct.
MongoDB Environment Setup and Connection
To begin automating MongoDB testing with Java, you'll need to set up your development environment. Here are the key components:
- Java Development Kit (JDK): Ensure you have JDK installed. You can download it from the official Oracle website.
- Maven: Apache Maven is a build automation tool used primarily for Java projects. It can manage project dependencies and build configurations. Download it from Maven's official site.
- MongoDB Java Driver: This library allows Java applications to connect to MongoDB databases. Add the dependency in your
pom.xml
:
<dependency>
<groupId>org.mongodb</groupId>
<artifactId>mongo-java-driver</artifactId>
<version>3.12.14</version>
</dependency>
- TestNG: A popular testing framework for Java. It is used to write and organize test cases, execute them efficiently, and generate detailed test reports. Add it to your
pom.xml
:
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.10.2</version>
</dependency>
Step-by-Step Guide to Test MongoDB Database
Testing a MongoDB database involves several steps. Here's how you can perform these steps using Java and TestNG. Below is a step-by-step guide with an example to test the creation and update operations in MongoDB and verify that the corresponding data is correctly stored in a MongoDB collection.
1. Test Class Initialization
The first step in our automation process is to set up the connection to MongoDB. We use the @BeforeClass
annotation to ensure that the setup method runs once before any tests are executed. In this setup method:
- We define the connection string to our MongoDB instance.
- We create
MongoClientSettings
using this connection string. - We instantiate a
MongoClient
and connect to the specified database and collection.
2. Test to Create Data inside MongoDB and Validate Data
This operation is encapsulated in the validateCreateDataTest
method, annotated with @Test
to signify that it is a test case. In this test method:
- We create a document with fields
name
,operation
, andage
. - We insert this document into the collection.
- We verify the insertion by querying the document and asserting the values.
- We store the document's
_id
for use in subsequent tests.
3. Test to Update Data inside MongoDB and Validate Data
After creating the document inside MongoDB, we need to test updating it. This is handled in the validateUpdateDataTest
method, which depends on the previous test. In this test method:
- We define new values for the document fields
name
andoperation
. - We create a query to find the document by its
_id
. - We create an update document with the new values.
- We update the document in the collection.
- We verify the update by querying the document and asserting the new values.
4. Tear Down Method
Finally, we need to clean up the database after all tests have run. This is done in the tearDown
method, annotated with @AfterClass
to ensure it runs after all test methods. In this method:
- We drop the database to remove all data created during the tests.
- We close the
MongoClient
to release resources.
These below are code snippet of Test Class which have been explained in above steps.
package com.test.automation;
import com.mongodb.ConnectionString;
import com.mongodb.MongoClientSettings;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
import org.bson.types.ObjectId;
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.Test;
import static com.generic.utilities.GenericUtil.getRandomNumber;
import static org.testng.AssertJUnit.assertEquals;
import static org.testng.AssertJUnit.assertNotNull;
public class MongoDBAutomationTest {
private static MongoClient mongoClient;
private static MongoDatabase database;
private static MongoCollection<Document> collection;
String name = "test-" + getRandomNumber(6);
Integer age = Integer.parseInt(getRandomNumber(2));
ObjectId id;
@BeforeClass
public void setUp() {
// Connection string (replace with your MongoDB URI)
String uri = "mongodb://localhost:27017"; // For local MongoDB instance
// Create a connection string and settings
ConnectionString connectionString = new ConnectionString(uri);
MongoClientSettings settings = MongoClientSettings.builder()
.applyConnectionString(connectionString)
.build();
// Create a MongoClient
mongoClient = MongoClients.create(settings);
// Access the database
database = mongoClient.getDatabase("testDatabase");
// Access the collection
collection = database.getCollection("testCollection");
}
@Test(priority=1)
public void validateCreateDataTest() {
String operation = "create";
// Insert a document
Document doc1 = new Document("name", name).append("operation", operation).append("age", age);
collection.insertOne(doc1);
// Verify the document was inserted
Document result = collection.find(new Document("name", name)).first();
assertEquals(result.getString("name"), name);
assertEquals(result.getString("operation"), operation);
assertEquals(result.getInteger("age"), age);
id = (ObjectId) result.get("_id");
assertNotNull(id);
}
@Test(priority=2,dependsOnMethods = {"validateCreateDataTest"})
public void validateUpdateDataTest() {
// Insert a document to update
String updated_name = "updated-" + name;
String operation = "update";
ObjectId objectId = new ObjectId(String.valueOf(id));
Document query = new Document("_id", objectId);
Document updatedDoc = new Document("$set", new Document("name", updated_name).append("operation", operation));
// Update the document
collection.updateOne(query, updatedDoc);
// Verify the document was updated
Document resultAfterUpdate = collection.find(query).first();
System.out.println("resultAfterUpdate: " + resultAfterUpdate.toJson());
assertEquals(resultAfterUpdate.getString("name"), updated_name);
assertEquals(resultAfterUpdate.getString("operation"), operation);
assertEquals(resultAfterUpdate.getInteger("age"), age);
}
@AfterClass
public void tearDown() {
// Drop the database after tests
database.drop();
// Close the MongoClient
mongoClient.close();
}
}
5. Report Generation
Here is the TestNG report generated for the two test methods. This report confirms that the tests for validating the creation and updating of test data in the MongoDB database have passed successfully with no failures or skipped tests.
Conclusion
Automation testing is a vital part of ensuring that your MongoDB applications perform reliably and efficiently. By using Java along with testing frameworks like TestNG, you can automate the testing of various database operations, ensuring data integrity, performance, and seamless integration into CI/CD pipelines. This approach not only enhances the quality of your application but also accelerates the development process by providing quick feedback on the health of your codebase.
References
- How we optimized the read operations using MongoDB at Halodoc
- Automation Testing
- Automated Sanity Suite Integration with Continuous Integration pipeline
Join Us
Scalability, reliability and maintainability are the three pillars that govern what we build at Halodoc Tech. We are actively looking for engineers at all levels, and if solving complex problems with challenging requirements is your forte, please reach out to us with your resumé at careers.india@halodoc.com.
About Halodoc
Halodoc is the number 1 all around Healthcare application in Indonesia. Our mission is to simplify and bring quality healthcare across Indonesia, from Sabang to Merauke. We connect 20,000+ doctors with patients in need through our Tele-consultation service. We partner with 3500+ pharmacies in 100+ cities to bring medicine to your doorstep. We've also partnered with Indonesia's largest lab provider to provide lab home services, and to top it off we have recently launched a premium appointment service that partners with 500+ hospitals that allow patients to book a doctor appointment inside our application. We are extremely fortunate to be trusted by our investors, such as the Bill & Melinda Gates Foundation, Singtel, UOB Ventures, Allianz, GoJek, Astra, Temasek, and many more. We recently closed our Series D round and In total have raised around USD$100+ million for our mission. Our team works tirelessly to make sure that we create the best healthcare solution personalised for all of our patient's needs, and are continuously on a path to simplify healthcare for Indonesia.