Automatically formatting and cleaning code on commit
Imagine yourself in a room. Do you prefer a tidy or a messy room? It would be easier to find your stuff in a clean room, and you would be more comfortable working there. The same goes for developers.
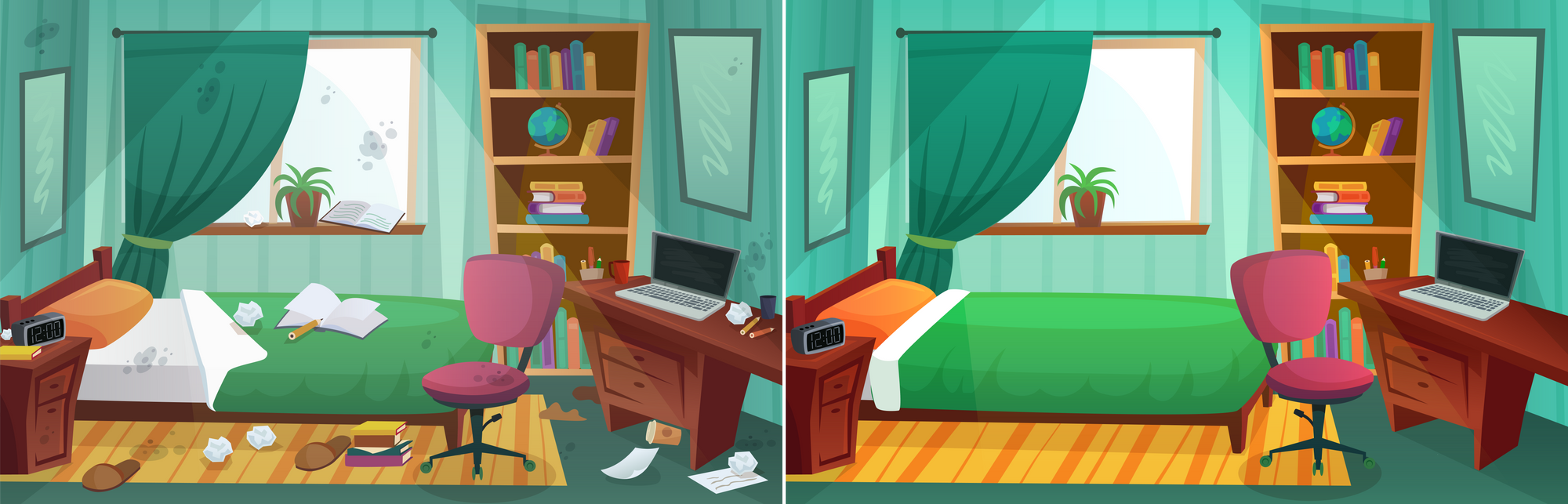
Every developer has their own coding style because they come from different backgrounds and institutions. To ensure everyone is on the same page about coding style and patterns, guidelines or rules are essential.
Why is it necessary to format and clean the code?
Let's say we don't have the same code style. If that happens, it will cause problems such as readability issues, developers taking more time to understand the code, bugs appearing, and when the codebase becomes more significant, it will be difficult to maintain. In the future, we can avoid the mess by cleaning up the code we want to commit to the repository. However, not every developer knows about the long-term code they build, especially when a deadline approaches and another task awaits. As a result, we created the formatter and cleaner in our code base to make the rules and run them automatically upon commit. Therefore, developers will have more time to maintain their code.
Our primary goal is to automatically format the CSS, HTML, and Typescript files. Here's how we do it 💫
Before then, we used several tools to add rules, create formats, and the tools to make them run when we committed the code.
- Linter: ESLint
Halodoc uses Typescript (Angular), so ESLint can find problems in our javascript and typescript files. A lot of problems found by ESLint can be corrected, more than that, we can customize the rules based on your needs of your company
Documentation: https://eslint.org/ - Code formatter: Prettier
Format the HTML, CSS, and TS file with the prettier rule. You will save time by not having to discuss style during code review.
Documentation: https://prettier.io/ - Git Hooks: Husky
Husky can improve our commits by using it to lint our commit messages and run ESLint and Prettier on pre-commit.
Documentation: https://typicode.github.io/husky/#/ - File stagger: Lint-staged
With this tool, husky only checks for the changes that developers made, so it does not contain all the files, only those that the developer made during the commit.
Documentation: https://www.npmjs.com/package/lint-staged
Install and setup the tools
Let's set up the tools and mix them!
Install the ESLint
Install ESLint using npm:
npm install eslint --save-dev
Then set up a configuration file:
npm init @eslint/config
As you set up the configuration file, you will find this question on your terminal. Fill this based on the configuration you prefer, and eslint will serve the configuration for you.
- What type of modules does your project use?
- Which framework does your project use?
- Where does your code run?
- How would you like to define a style for your project?
- What format do you want your config file to be in?
Having answered all of the questions, you will have a .eslintrc.js file in your root folder, and you're ready to move on.
*When you want a fresh start without overanalyzing the rules, you just remove all of the rules in .eslintrc.js, and the default rule of eslint will be setup.
module.exports = {
env: {
browser: true,
node: true,
es2021: true,
},
extends: ["eslint:recommended", "plugin:@typescript-eslint/recommended"],
parser: "@typescript-eslint/parser",
parserOptions: {
ecmaVersion: "latest",
sourceType: "module",
},
plugins: ["@typescript-eslint"],
rules: {},
};
Install Prettier
Install using npm command:
npm install prettier --save-dev
You don't have to config anything here. Just install and go to the next step
Install Lint Staged
Install using npm command:
npm install lint-staged --save-dev
You don't have to config anything here. Just install and go to the next step
Install Husky
Install using npm command:
npm install husky --save-dev
If you experience the error "could not determine executable to run" when committing your code, you need to reinstall husky this command or remove your node_modules and reinstall node
npm uninstall husky && npm install --save-dev husky
add this config in package.json
"lint-staged": {
"src/**/*.{html,ts,scss}": [
"prettier --write"
],
"src/**/*.ts": [
"eslint --fix"
]
},
"husky": {
"hooks": {
"pre-commit": "lint-staged"
}
}
This code will run pre-commit when the developer commits the changes. The pre-commit will automatically call the prettier --write
to format the HTML, CSS, and TS file and call eslint --fix
to check the TS file rules.
Run this command to create a shell script husky
npm set-script prepare "husky install"
npm run prepare
After that, create a pre-commit file by running this command. This will call the lint-staged in package.json
npx husky add .husky/pre-commit "npx lint-staged"
Commit all of the files, and you are ready to go.
That's it. Now you can automate the formatting and cleaning your code at commit time.
See the demo 💫
Let's give it a try!
Format CSS
This sample code shows that CSS file is not clean, and when i run commit, the CSS file is formatted.
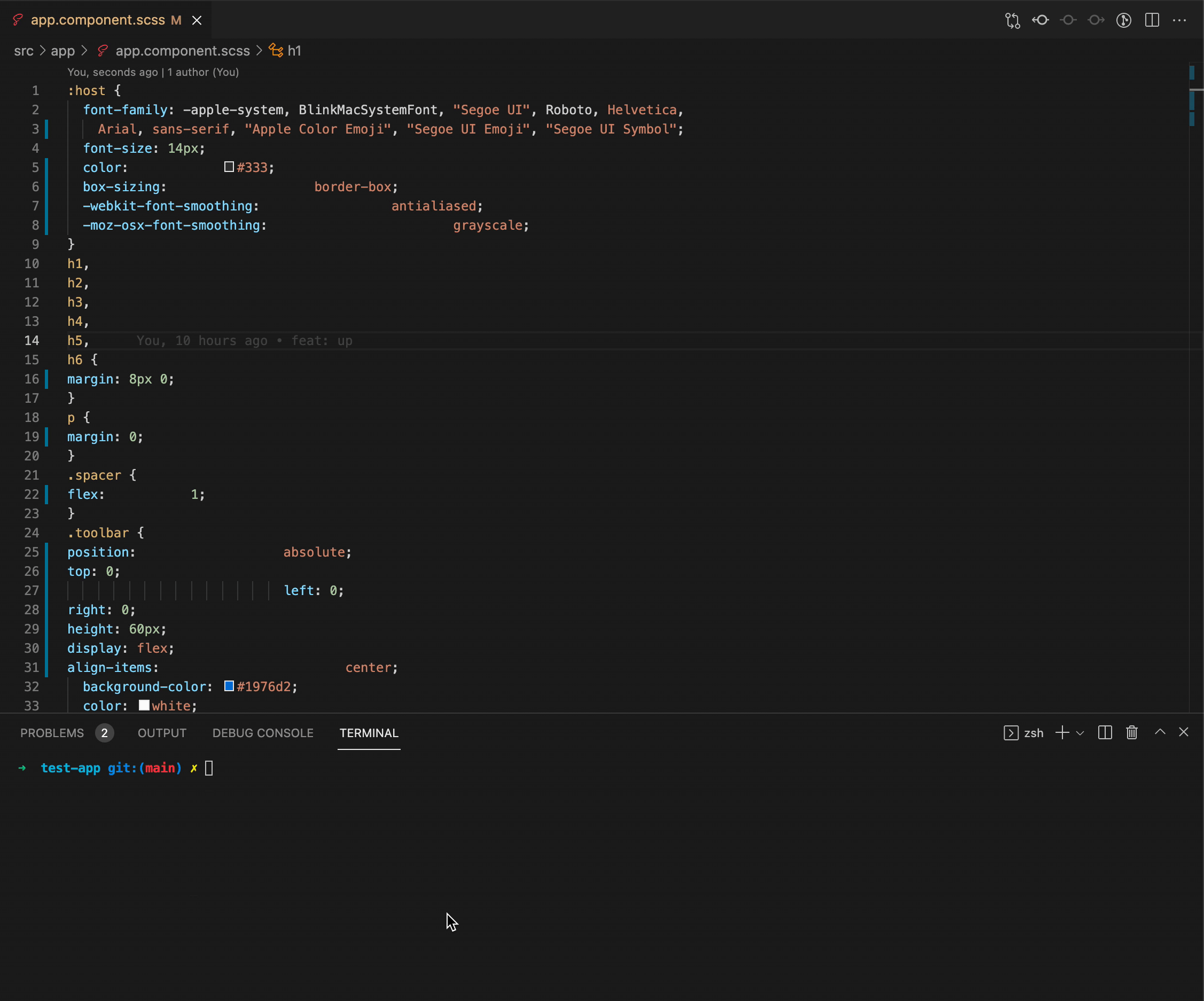
Format HTML
HTML files where automatically formatted when committing , just like CSS files
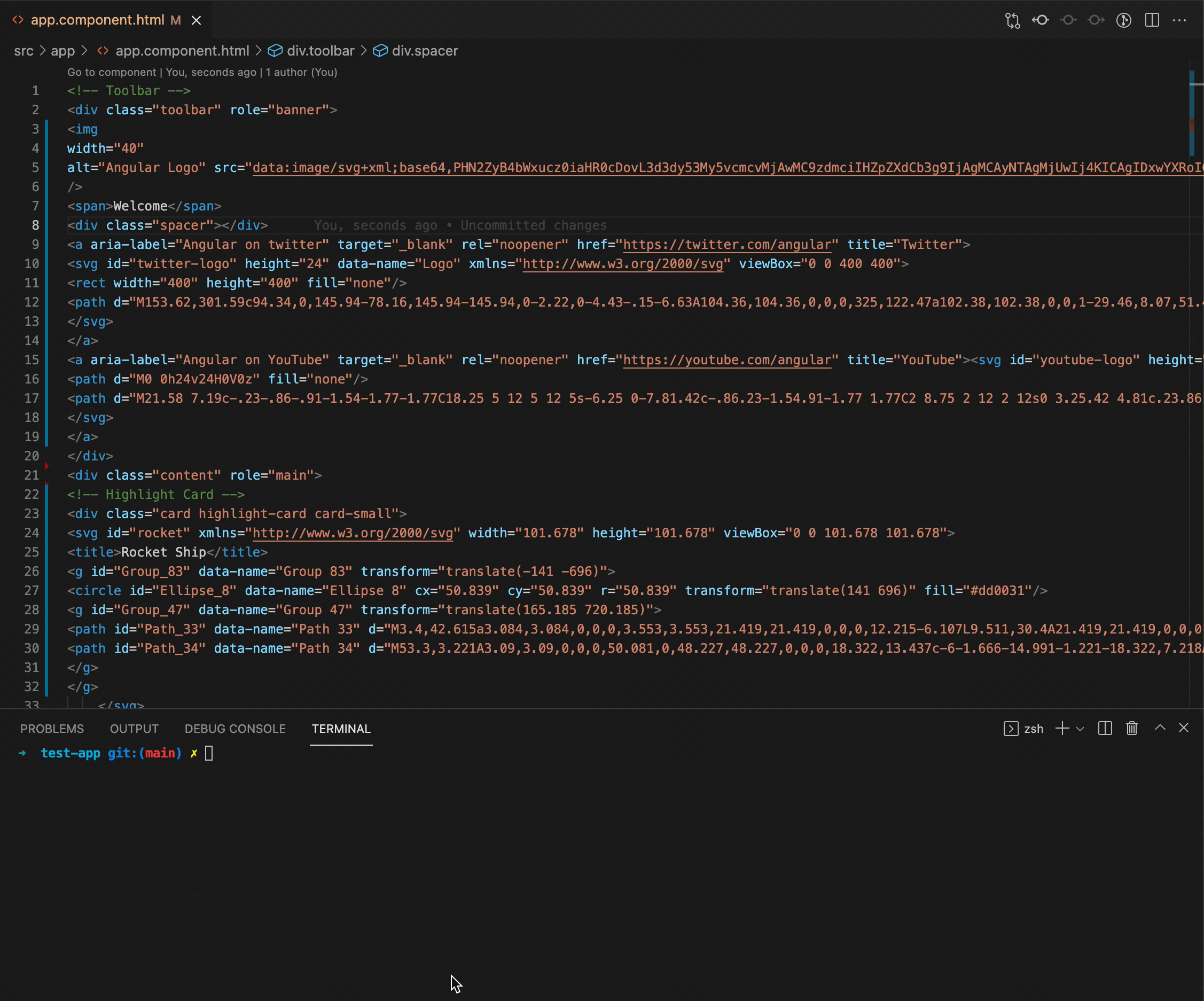
Format TS
Just like CSS and HTML, but this one is Typescript. It automatically formats when it committed.
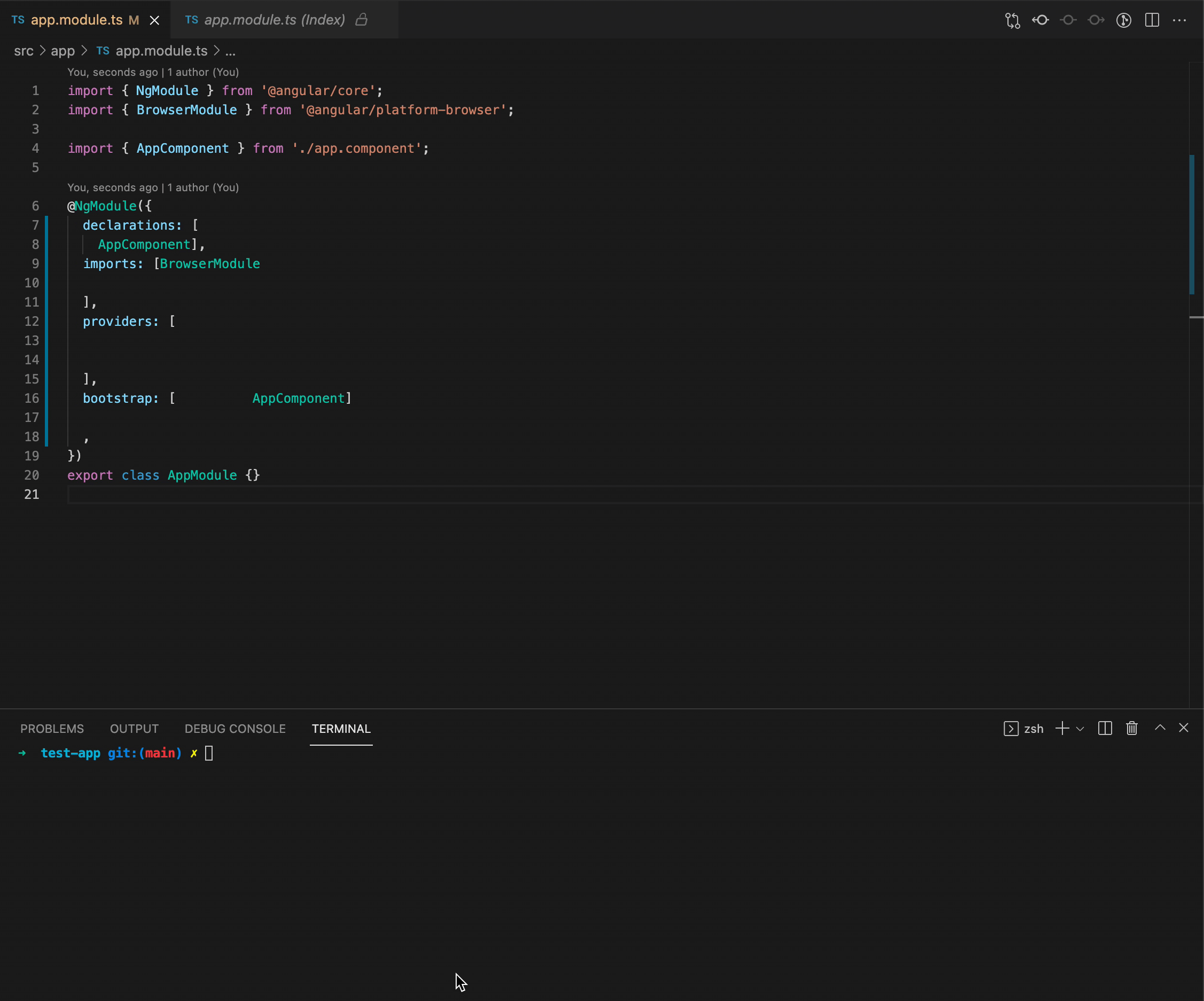
Find problems in TS and throw errors
Another advantage is that we can detect and throw errors on Typescript based on the syntax we use
For example, adding the type Object is not good because it means any non-null value
, or we can detect unused variables, such as 'a' is assigned value but is never used
. These findings allows us to update our code and removed the unused variables we forgot to remove when developing
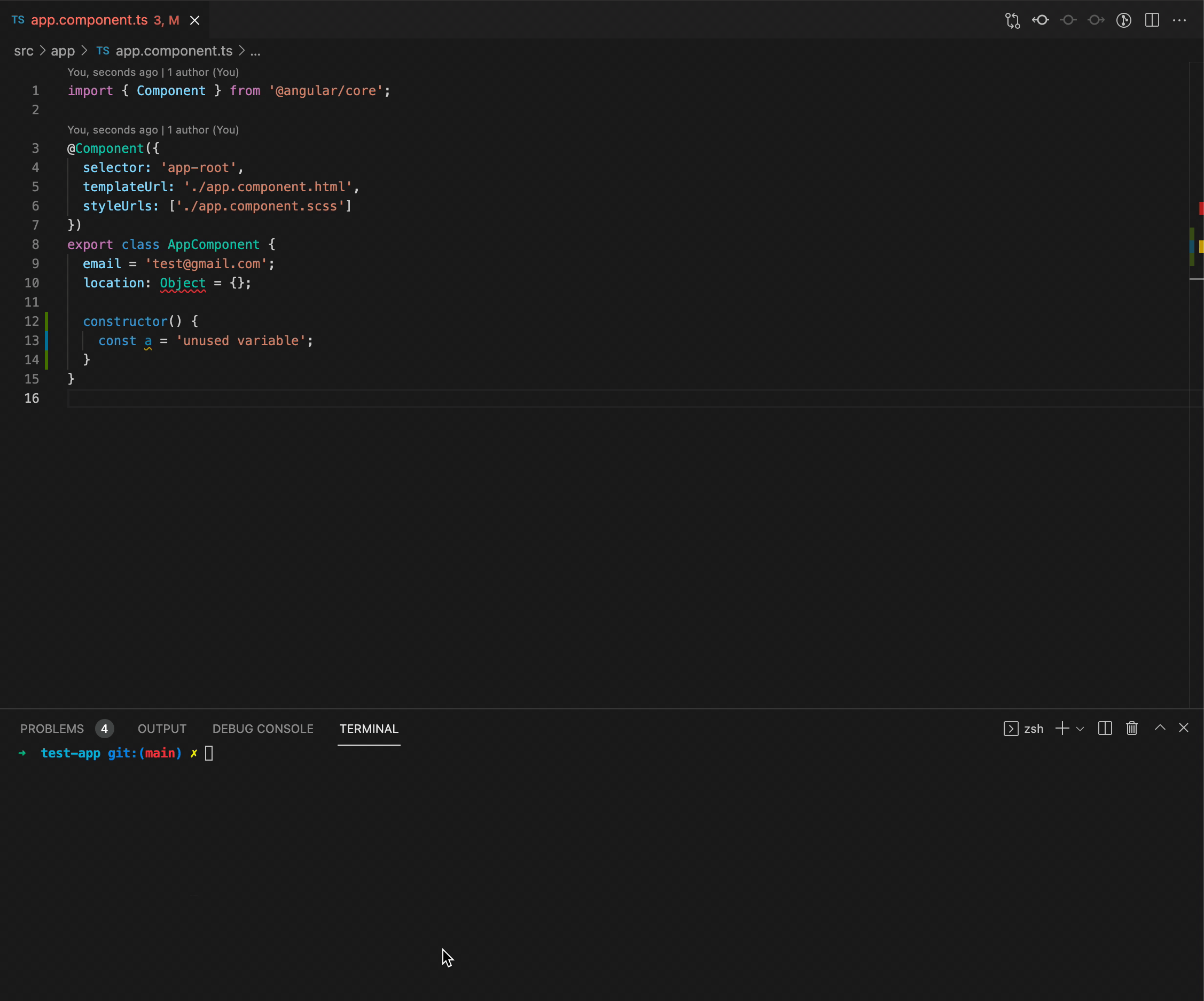
Summary
We hear about user experience because we want our users to be comfortable and keep using our app. However, on the backside developers also build the app. We need to take the developer experience into consideration as well so that they are happy. A key aspect of this automatically cleaning up our code.
By using automated linter and code formatting, code review and coding can be accelerated . Having logically formatted and well-structured source code greatly improves bug hunting and feature enhancements. Additionally, readability and maintenance issues are resolved, allowing developers to focus on solving actual problems.
References:
- Prettier documentation: https://prettier.io/
- ESLint documentation: https://eslint.org/
- Husky documentation: https://typicode.github.io/husky/#/
- Lint Staged documentation: https://www.npmjs.com/package/lint-staged
Join us
Scalability, reliability and maintainability are the three pillars that govern what we build at Halodoc Tech. We are actively looking for engineers at all levels and if solving hard problems with challenging requirements is your forte, please reach out to us with your resumé at careers.india@halodoc.com.
About Halodoc
Halodoc is the number 1 all around Healthcare application in Indonesia. Our mission is to simplify and bring quality healthcare across Indonesia, from Sabang to Merauke. We connect 20,000+ doctors with patients in need through our Tele-consultation service. We partner with 3500+ pharmacies in 100+ cities to bring medicine to your doorstep. We've also partnered with Indonesia's largest lab provider to provide lab home services, and to top it off we have recently launched a premium appointment service that partners with 500+ hospitals that allow patients to book a doctor appointment inside our application. We are extremely fortunate to be trusted by our investors, such as the Bill & Melinda Gates Foundation, Singtel, UOB Ventures, Allianz, GoJek, Astra, Temasek and many more. We recently closed our Series C round and In total have raised around USD$180 million for our mission. Our team works tirelessly to make sure that we create the best healthcare solution personalized for all of our patient's needs, and are continuously on a path to simplify healthcare for Indonesia.