Modernising iOS Apps: The Benefits and Challenges of Moving from XIBs to SwiftUI
SwiftUI is Apple’s modern UI framework introduced at WWDC 2019, designed to simplify user interface development across all its platforms. Unlike UIKit, which requires manual management of UI updates, SwiftUI lets developers define the desired outcome while the system automatically handles updates and state changes. It also integrates seamlessly with Swift’s advanced features, such as Combine and Swift Concurrency, to create more responsive and efficient apps.
Since its introduction in iOS 13, SwiftUI has continuously evolved, adding new components, enhancing performance, and expanding platform compatibility. While it hasn't fully replaced UIKit, Apple's continued investment in SwiftUI reinforces its significance as the future of UI development.
Transitioning an existing UIKit-based app to SwiftUI comes with challenges, such as incomplete feature parity and compatibility with existing UIKit code. In this blog, we’ll explore why adopting SwiftUI is beneficial, the obstacles developers may face, and how to overcome them. Whether you're planning a full transition or a gradual integration, understanding these factors will help streamline the process.
Why Consider Migrating to SwiftUI?
Migrating to SwiftUI offers several advantages that can enhance your app’s development, performance, and maintainability. Apple continues to prioritize SwiftUI, introducing new features and deeper system integrations, making it the future of UI development. Unlike UIKit, SwiftUI simplifies UI creation with less boilerplate code, reducing development time and improving readability. Its declarative syntax makes managing complex UIs more intuitive, while built-in state management with @State
, @Binding
, and @ObservableObject
ensures a more responsive user experience.
To illustrate how much simpler UI creation can be with SwiftUI, let's compare a common UI element—a simple list—implemented in both frameworks. In UIKit, you'd typically set up a UITableView
, manage its data source and delegate methods, and configure cell reuse either programmatically or via XIBs. In contrast, SwiftUI's declarative syntax allows you to build the same list with just a few lines of code. This example offers a clear look at the distinct development styles of the two frameworks.
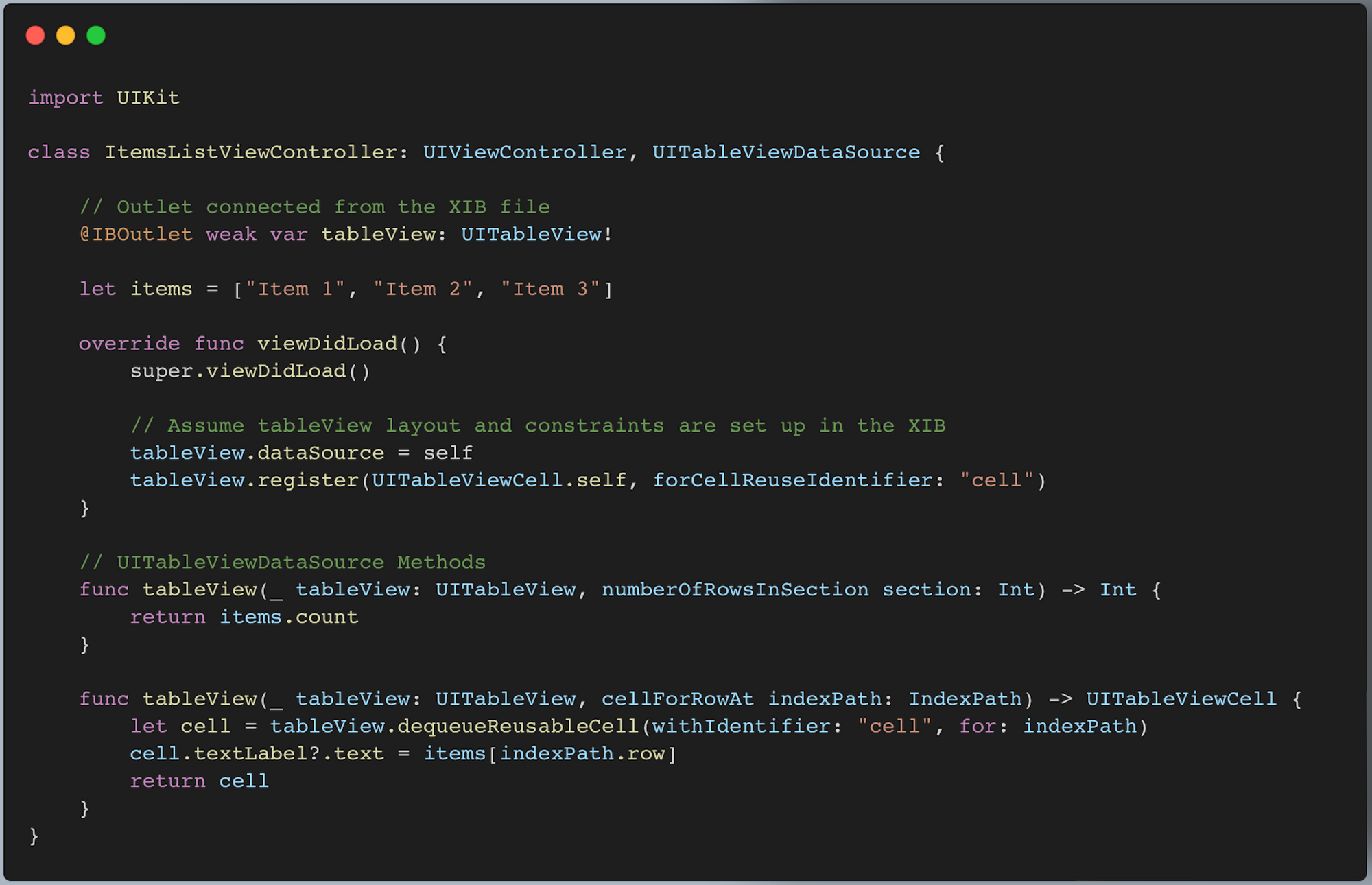
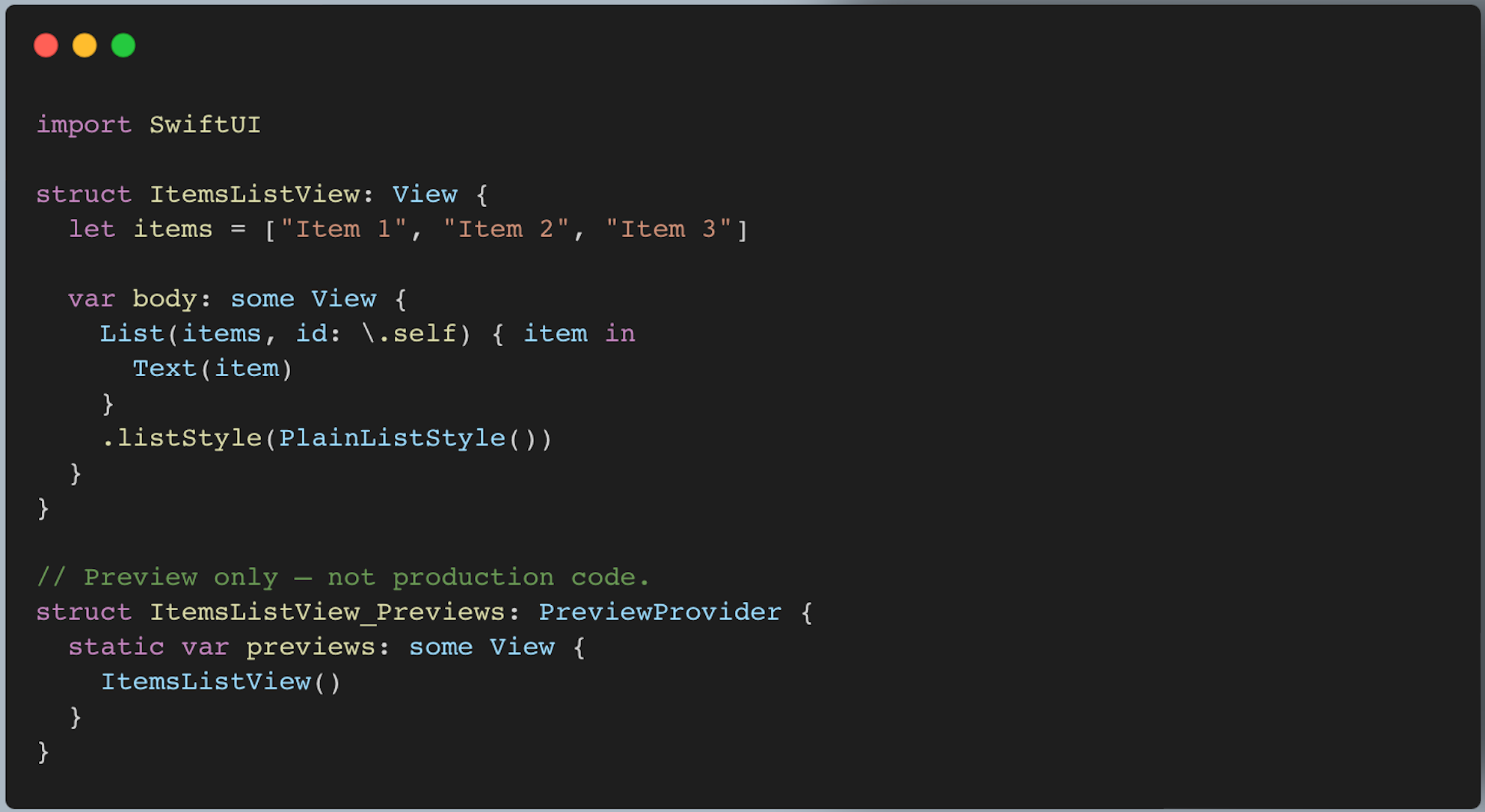
Below are some key differences in how a simple list is constructed in UIKit and SwiftUI:
- Code Structure: UIKit uses an imperative approach that requires manually setting up views, constraints, and delegate methods. In contrast, SwiftUI’s declarative syntax lets you define the entire UI in a concise view hierarchy.
- Conciseness: UIKit implementations often involve extra boilerplate code, while SwiftUI achieves similar results with significantly fewer lines.
- Layout Management: In UIKit, you manage layouts using Auto Layout or XIB configurations. SwiftUI automatically handles layout based on how you compose your views.
- Live Feedback: SwiftUI integrates directly with Xcode Previews for real-time visual updates, unlike UIKit, which typically requires a full build and run to see changes.
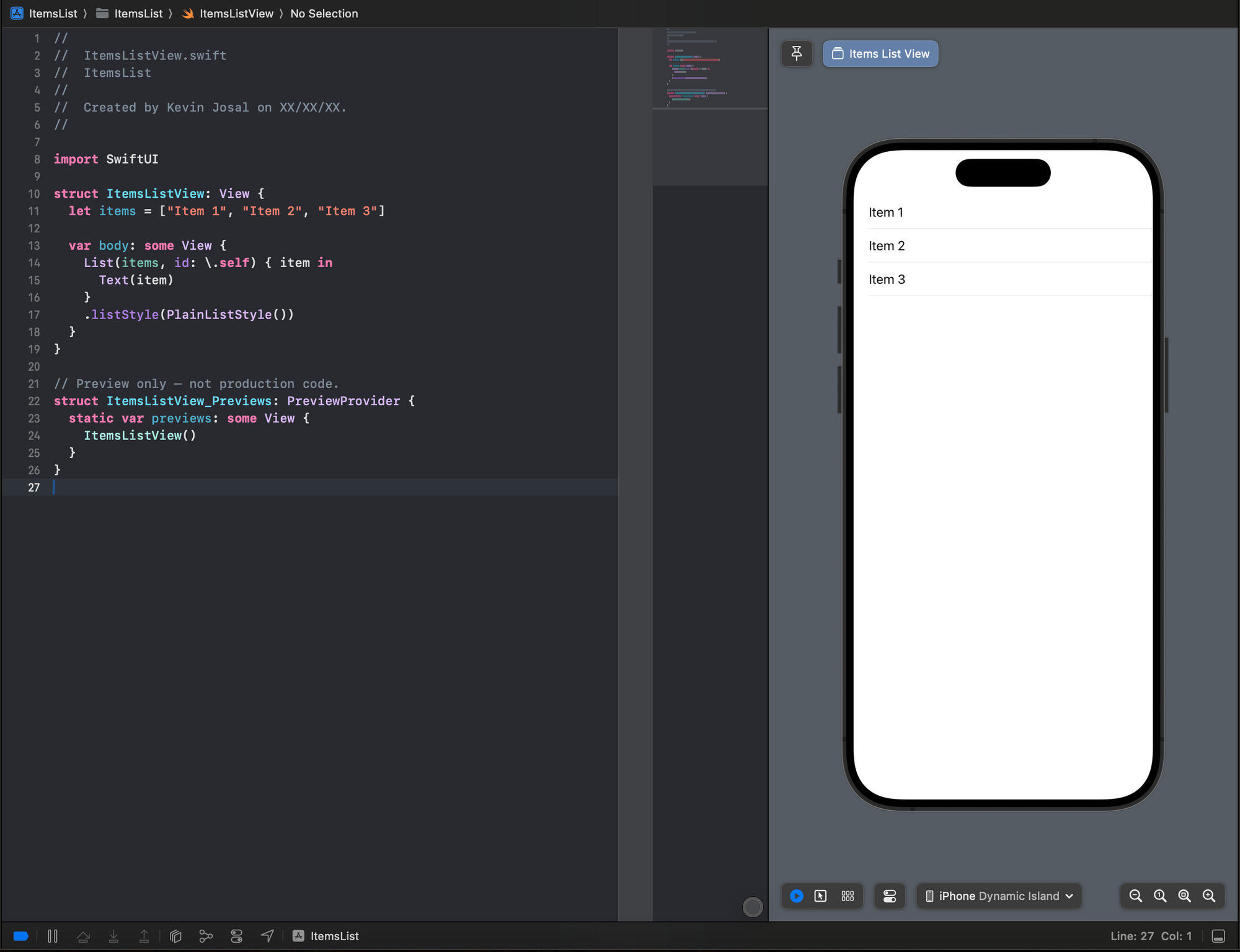
Additionally, Xcode Previews support testing across multiple device sizes, color schemes, and dynamic text settings simultaneously, ensuring a consistent and adaptable user experience. While occasional refresh issues may occur, the increased development speed and smoother workflow make it an invaluable tool for modern UI design.
Challenges of Migrating from UIKit to SwiftUI
While SwiftUI offers many benefits, transitioning from UIKit presents several challenges that developers must navigate:
1. Incomplete Feature Parity
One of the biggest challenges when transitioning to SwiftUI is the lack of full feature parity with UIKit. While SwiftUI continues to evolve, certain advanced capabilities and customization options available in UIKit do not yet have direct equivalents. This can create roadblocks for developers who rely on complex UIKit components. Below are some of the examples:
- Rich Text Display:
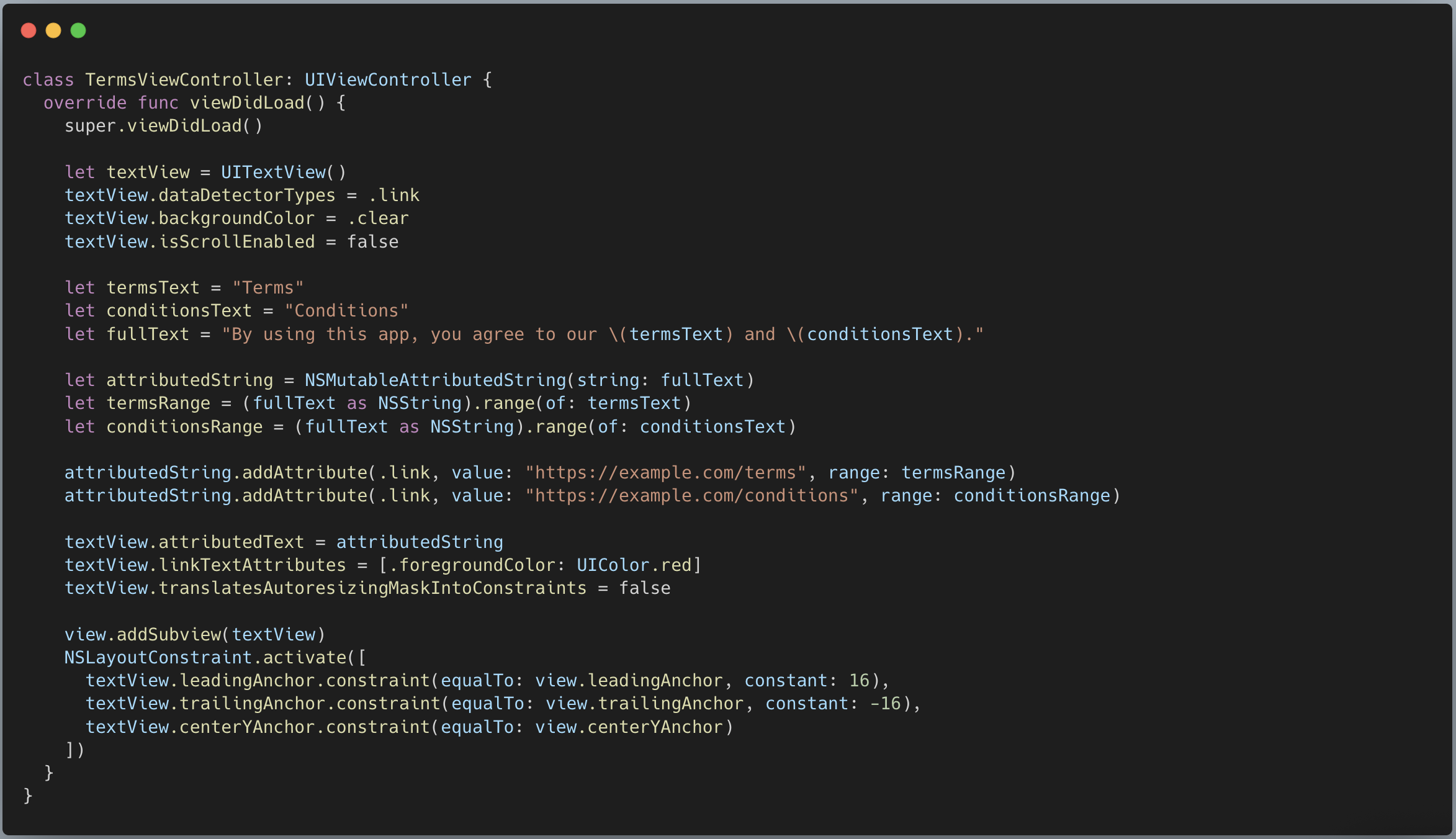
UIKit’s UITextView
fully supports rich text formatting using NSAttributedString
, allowing developers to style text with different colors, hyperlinks, bold, and underlines. However, SwiftUI’s TextEditor
and Text
lack support for rich text editing, limiting developers who need more advanced formatting capabilities.
- iOS 15+ Improvement: SwiftUI introduced support for
AttributedString
, allowing developers to style text with different attributes. This brings SwiftUI closer to UIKit but still lacks some advanced features, such as interactive text selection and dynamic formatting within editable text fields.
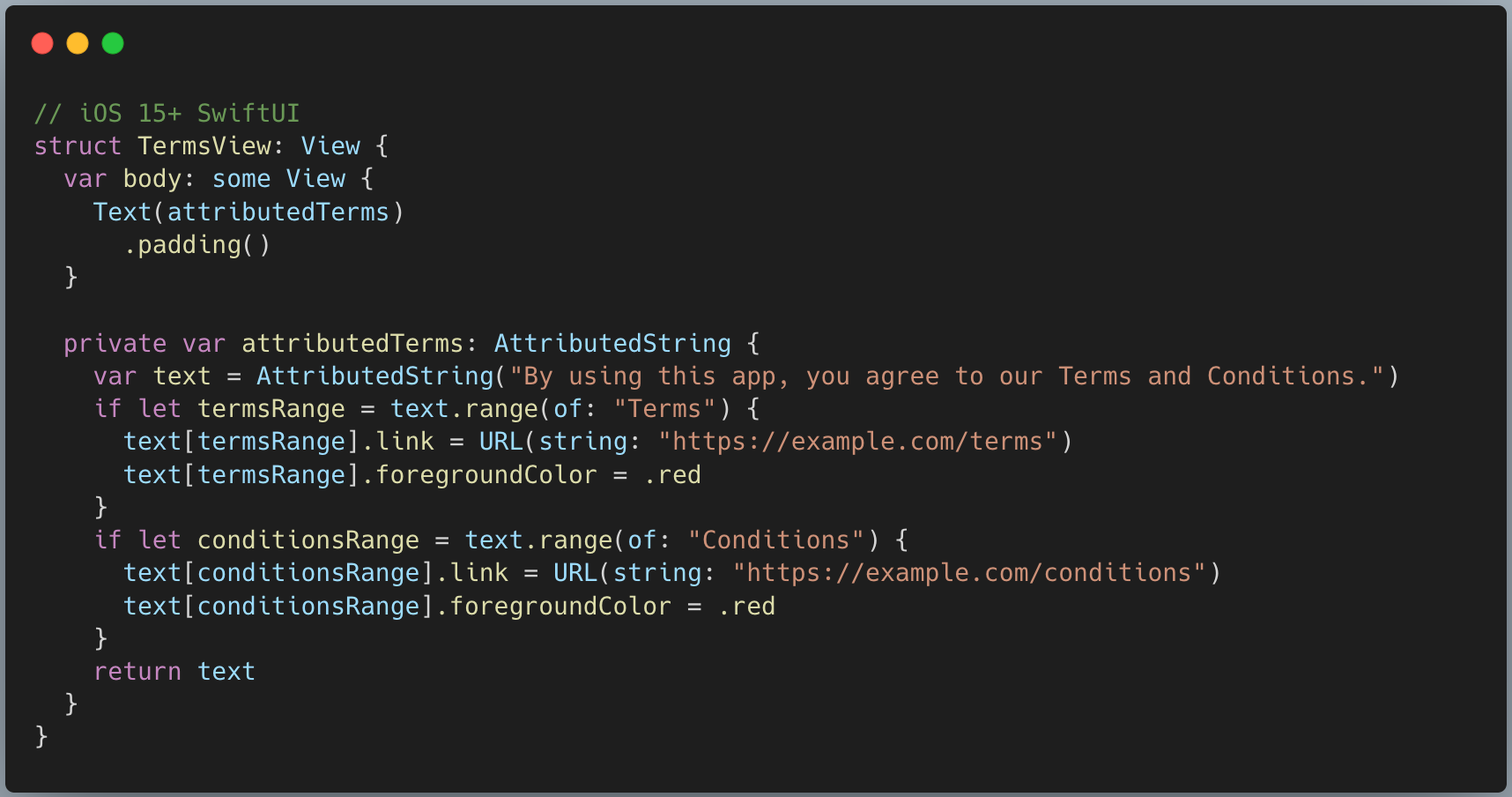
- iOS 14 and Below Workaround: Since SwiftUI’s
Text
does not support rich text formatting in earlier versions, a common workaround is embeddingUITextView
inside SwiftUI usingUIViewControllerRepresentable
.
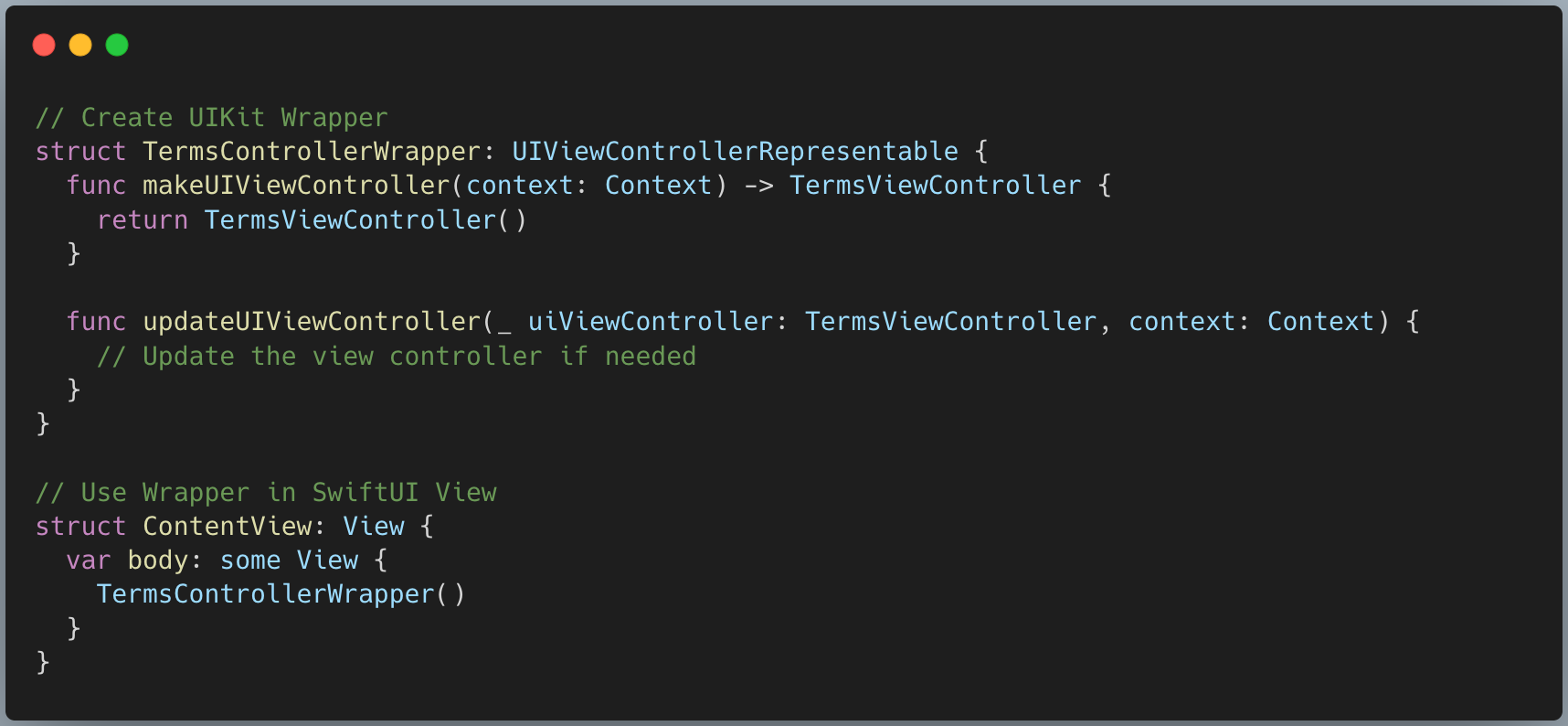
- Grid
When SwiftUI was first introduced in iOS 13, it lacked a built-in grid system—a feature that UIKit’s UICollectionView
had long provided. As a result, creating grid layouts in SwiftUI was challenging. Developers had to manually construct grids using a combination of HStack
and VStack
, carefully distributing elements into rows and columns. While this approach was functional, it was far from efficient.
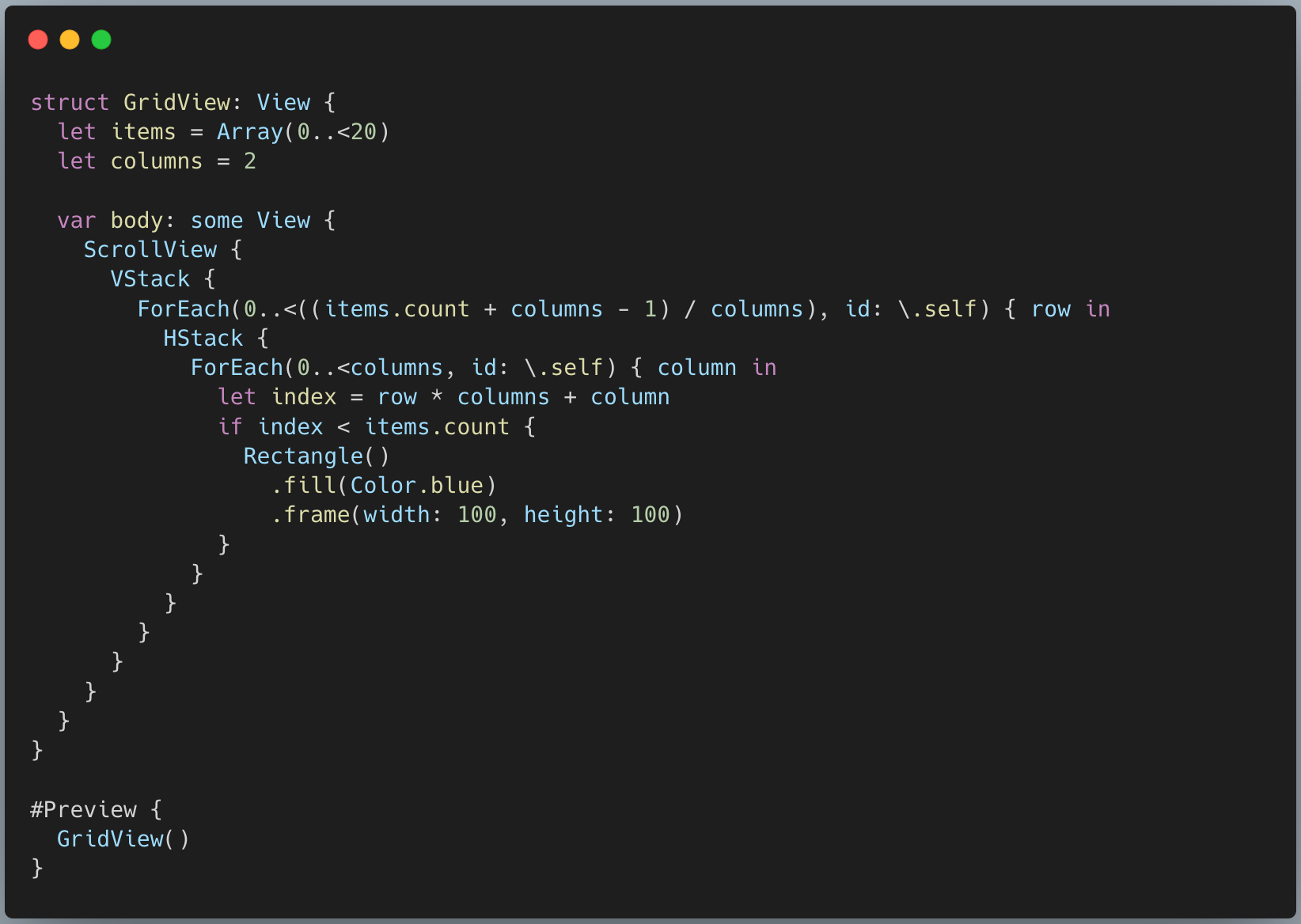
Finally, in iOS 14, SwiftUI introduced LazyVGrid
, bringing long-awaited support for grid layouts. Unlike the manual and cumbersome approach of iOS 13, LazyVGrid
provided a declarative, efficient way to structure content. It automatically handled row and column distribution and optimized performance by rendering only visible items.
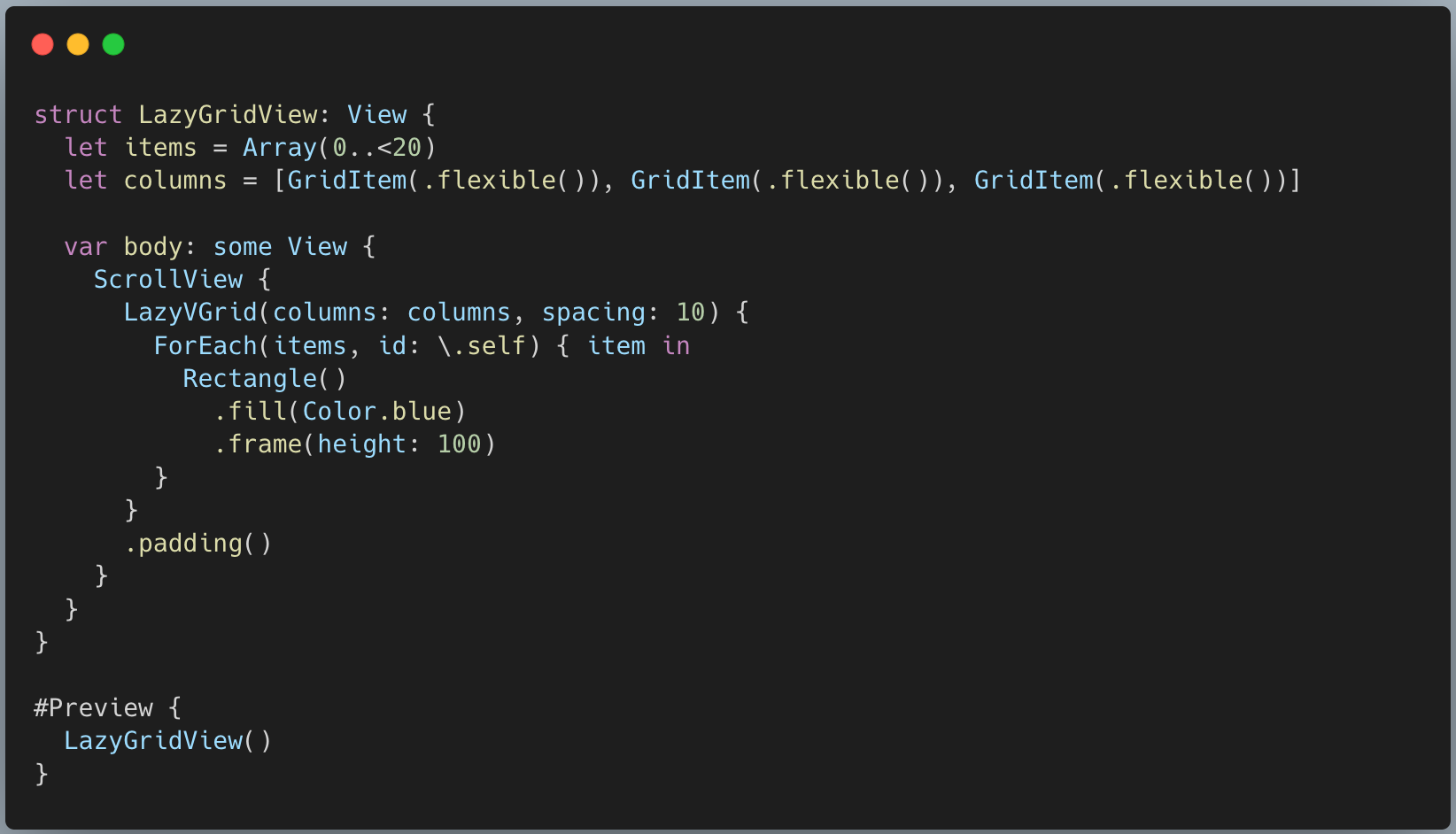
These are just a few examples of the gaps in SwiftUI’s feature set compared to UIKit. While SwiftUI has made significant progress, certain areas still lack the depth and flexibility that UIKit provides. Developers often find themselves needing workarounds or even falling back to UIKit for advanced functionality.
2. Compatibility with Existing UIKit Code
Most UIKit-based apps rely on mature, well-tested codebases. A full transition to SwiftUI isn’t always practical, which is why a gradual integration is often the preferred strategy. Bridging SwiftUI and UIKit within the same project can add complexity, as developers need to manage interoperability between the two frameworks.
3. Platform Version Limitations
SwiftUI is only fully available on iOS 13 and later, with many key improvements introduced in newer versions. Supporting older iOS versions may require maintaining UIKit-based implementations alongside SwiftUI, increasing development overhead.
Despite these challenges, SwiftUI continues to improve with each release, making gradual adoption a viable strategy. By understanding these limitations and planning accordingly, developers can make the transition smoother and future-proof their apps.
Migration Strategies: Full and Gradual Integration
Migrating from UIKit to SwiftUI requires a well-planned approach to balance modernization with maintainability. Depending on your project’s scope, you can choose between a full transition or a gradual integration.
Full Transition: Rebuilding the App in SwiftUI
A full transition involves rewriting the entire UI using SwiftUI, replacing UIKit completely. This approach is best suited for:
- New projects that can be built entirely in SwiftUI from the start.
- Apps with outdated UIKit codebases that require significant refactoring.
- Projects targeting only newer iOS versions (iOS 13+), minimizing backward compatibility concerns.
Gradual Integration: Introducing SwiftUI into an Existing UIKit App
A gradual integration allows developers to introduce SwiftUI step by step without fully replacing UIKit. This strategy is ideal for:
- Large, established apps where a full rewrite is impractical.
- Teams new to SwiftUI looking for a smoother learning curve.
- Apps that need to maintain compatibility with older iOS versions.
Common Approaches for Gradual Integration:
- Embedding SwiftUI Views in UIKit: Use
UIHostingController
to integrate SwiftUI views within UIKit-based screens. - Using
UIViewControllerRepresentable
: Wrap existing UIKit view controllers inside SwiftUI usingUIViewControllerRepresentable
to retain complex functionalities that are not yet available in SwiftUI. - Replacing Individual Screens: Migrate isolated features or new sections to SwiftUI while keeping the rest in UIKit.
- Adopting SwiftUI for New Features: Implement only newly designed features in SwiftUI while maintaining UIKit for legacy parts.
Choosing the Right Strategy
The best migration approach depends on your app’s size, complexity, and future goals. While a full transition offers a cleaner long-term solution, a gradual integration minimizes risk and disruption. In many cases, starting with gradual integration is the most practical path, allowing teams to adopt SwiftUI without compromising stability.
Conclusion
Migrating from UIKit to SwiftUI is a strategic decision that requires careful planning but offers long-term benefits in development efficiency, maintainability, and performance. While SwiftUI is still evolving, Apple’s continued investment in the framework reinforces its role as the future of UI development across all Apple platforms.
For new projects or major rewrites, a full transition to SwiftUI provides a clean, modern architecture. However, for existing UIKit apps, a gradual integration—leveraging UIHostingController
or UIViewControllerRepresentable
to bridge the gap—minimizes disruption while embracing SwiftUI’s advantages step by step.
Despite challenges such as incomplete feature parity and compatibility issues with existing UIKit code, SwiftUI offers simplified UI creation, enhanced state management, and powerful real-time previews. By adopting a gradual, phased approach, developers can incrementally modernize their apps while ensuring stability. Starting with small, manageable components and integrating SwiftUI over time is a forward-thinking strategy that aligns with Apple’s evolving ecosystem and keeps your app future-proof.
References
Join us
Scalability, reliability, and maintainability are the three pillars that govern what we build at Halodoc Tech. We are actively looking for engineers at all levels, and if solving hard problems with challenging requirements is your forte, please reach out to us with your resume at careers.india@halodoc.com.
About Halodoc
Halodoc is the number 1 Healthcare application in Indonesia. Our mission is to simplify and bring quality healthcare across Indonesia, from Sabang to Merauke. We connect 20,000+ doctors with patients in need through our Tele-consultation service. We partner with 3500+ pharmacies in 100+ cities to bring medicine to your doorstep. We've also partnered with Indonesia's largest lab provider to provide lab home services, and to top it off we have recently launched a premium appointment service that partners with 500+ hospitals that allow patients to book a doctor appointment inside our application. We are extremely fortunate to be trusted by our investors, such as the Bill & Melinda Gates Foundation, Singtel, UOB Ventures, Allianz, GoJek, Astra, Temasek, and many more. We recently closed our Series D round and in total have raised around USD$100+ million for our mission. Our team works tirelessly to make sure that we create the best healthcare solution personalized for all of our patient's needs, and are continuously on a path to simplify healthcare for Indonesia.