Building Beyond Boundaries: A Developer's Guide to Crafting Custom SDKs in Java
In the dynamic world of software development, tools and frameworks are key to project success. Among these, Software Development Kits (SDKs) are indispensable for streamlining and simplifying the development process. This blog explores Java SDKs, offering an overview, understanding of their significance, real-world success stories and crafting your own custom SDK.
Overview
Recognizing the importance of SDKs is crucial for both developers and businesses. At Halodoc, we heavily use SDKs to streamline our development. This blog delves into the intricacies of SDKs, offering insights into their nature. Additionally, we embark on creating our own SDK for real-life use cases, showcasing the practicality and versatility of these tools.
What is an SDK?
A software development kit (SDK) is a set of platform-specific building tools for developers. You require components like debuggers, compilers, and libraries to create code that runs on a specific platform, operating system, or programming language. SDKs put everything you need to develop and run software in one place. Additionally, they contain resources like documentation, tutorials, and guides as well as APIs and frameworks for faster application development.
Why Use SDKs?
SDKs offer several benefits across the development process that help developers create applications. They include:
- Efficiency and Time Savings: SDKs encapsulate commonly used functionalities, reducing the need for developers to write code from scratch. This accelerates the development process, allowing teams to focus on core application logic rather than repetitive tasks.
- Consistency and Standardization: SDKs provide a standardized set of tools and libraries, ensuring consistency across different projects. This not only simplifies the development process but also enhances maintainability and collaboration within development teams.
- Access to Platform Features: SDKs offer developers access to platform-specific features and services, enabling them to leverage the full potential of the underlying technology. This is particularly evident in the case of Java SDKs, which allow seamless integration with the Java Virtual Machine (JVM) and the Java Runtime Environment (JRE).
- Community Support and Updates: Popular SDKs often have vibrant developer communities that contribute to ongoing improvements, updates, and bug fixes. Utilizing an SDK with strong community support ensures that developers have access to valuable resources and assistance when facing challenges.
- Cost savings: SDKs reduce the time and resources needed to develop applications. By providing a library of pre-built components and tools, SDKs enable developers to quickly build out features and functionality. SDKs reduce the time and costs required to create new applications. They also reduce costs associated with deploying and maintaining applications, providing simplified installation processes and updates.
- Centralized Maintenance: Centralizing bug fixes, feature enhancements, and maintenance makes the process more straightforward. This ensures that all users of the SDK can easily access updates and improvements without the need for individual, scattered implementations.
Real-world Examples of Java SDK Success Stories
- Amazon Web Services (AWS) SDK for Java: AWS offers a comprehensive SDK for Java, allowing developers to seamlessly integrate Java applications with various AWS services. This SDK has played a pivotal role in the success of countless cloud-based applications, providing scalable and reliable solutions.
- Google Cloud Client Library for Java: Google Cloud's SDK for Java facilitates the integration of Java applications with Google Cloud services. This SDK has been instrumental in the development of applications ranging from data analytics to machine learning, showcasing the versatility of Java in cloud-based environments.
- Twilio Java SDK: Twilio's Java SDK empowers developers to integrate communication features, such as SMS, voice, and video, into their applications. This SDK has been widely adopted in sectors like healthcare, finance, and e-commerce, enhancing customer engagement and communication.
Internal Halodoc SDKs
- Halodoc-kafka SDK: This SDK streamlines the integration of Kafka-related processes within our internal microservices architecture. It manages tasks such as publishing and consuming messages. In the Halodoc backend, which is structured around microservices, the Kafka SDK plays a pivotal role in enabling an event-driven system.
- Halodoc-commons SDK: This versatile SDK within the Halodoc ecosystem takes charge of a variety of functionalities, including handling HTTP utilities, managing JPA-related workflows and providing internal utility classes. It serves as a comprehensive toolkit, streamlining common tasks and promoting efficiency across diverse aspects of our system architecture. The Halodoc-Commons SDK is designed to enhance development processes by offering a centralized and cohesive set of tools for a range of functionalities crucial to our application infrastructure.
- Halodoc-Schema SDK: This essential toolkit at Halodoc serves as a robust solution for the generation of Protobuf schemas. With a focus on efficiency and precision, the Halodoc-Schema SDK facilitates seamless schema generation, contributing to the optimization and standardization of data structures within our systems. This SDK plays a crucial role in ensuring compatibility and consistency across various components, enhancing the overall resilience and coherence of our data-driven workflows.
SDK vs API
While an SDK and an API are related, they serve different purposes. An SDK is used to create applications for a specific platform or programming language. An SDK typically includes an API, but it also includes other resources like documentation, sample code, and development tools.
On the other hand, an API is a set of protocols, routines, and tools that specify how software components should interact with each other. An API provides a way for developers to access specific features or services of a system or application. APIs can be used to communicate with software libraries, web services, and operating systems.
Building your custom SDK
Creating a custom SDK, such as a Kafka SDK for seamless event publishing and consumption, involves several steps. Below is a step-by-step guide with sample code for each step:
What is kafka?
- Apache Kafka is an open-source distributed event streaming platform used by thousands of companies for high-performance data pipelines, streaming analytics, data integration, and mission-critical applications.
Step 1: Define the Purpose and Scope of the SDK
Clearly define the purpose and scope of your SDK. In this case, we're creating a Kafka SDK for publishing and consuming events.
Step 2: Set Up Your Project
Create a new project for your SDK. You can use a build tool like Maven to manage dependencies and build configurations.
Step 3: Add Dependencies
Add the necessary dependencies to your project. For a Kafka SDK, you'll need the Kafka client library . Here's an example using Maven:
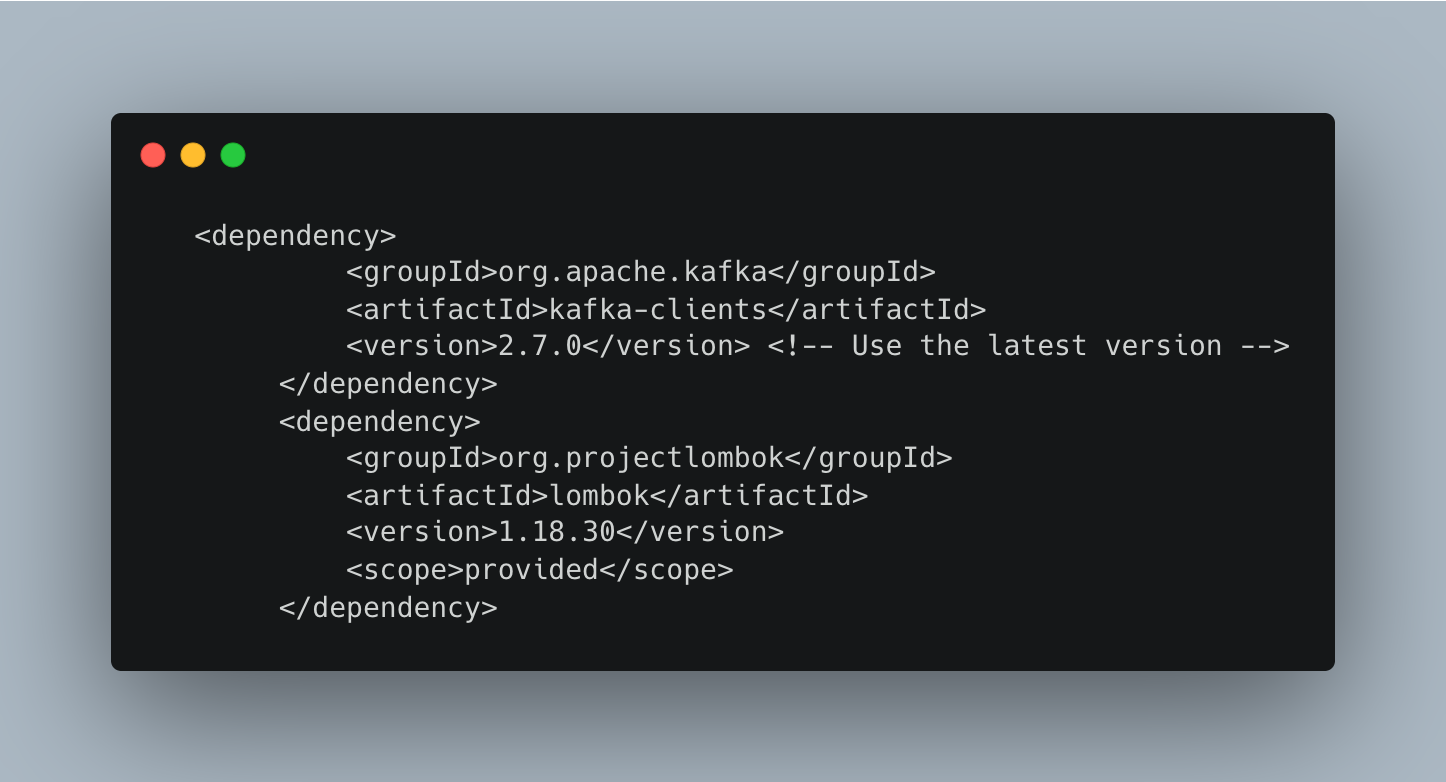
Step 4: Implement Producer and Consumer Classes
Create classes for the Kafka producer and consumer. Below are simplified examples:
Configuration Class:
- In this class, we are specifying the configurations necessary for integrating with Kafka.
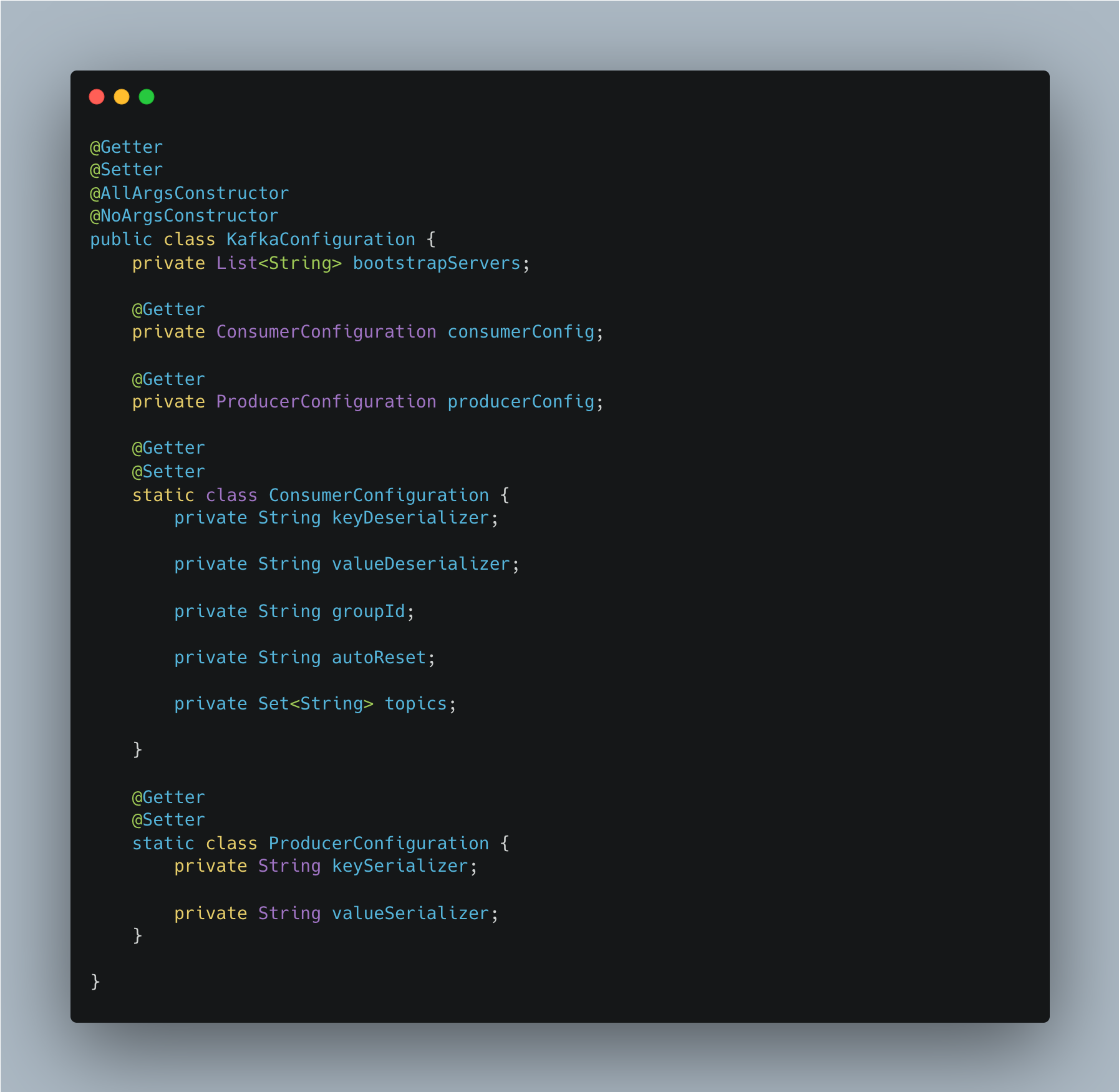
Producer Class:
- We utilize the
publishEvent()
method to dispatch events to Kafka topics. Internally, within this method, we employ theproducer.send()
method to transmit records to the designated Kafka topic.
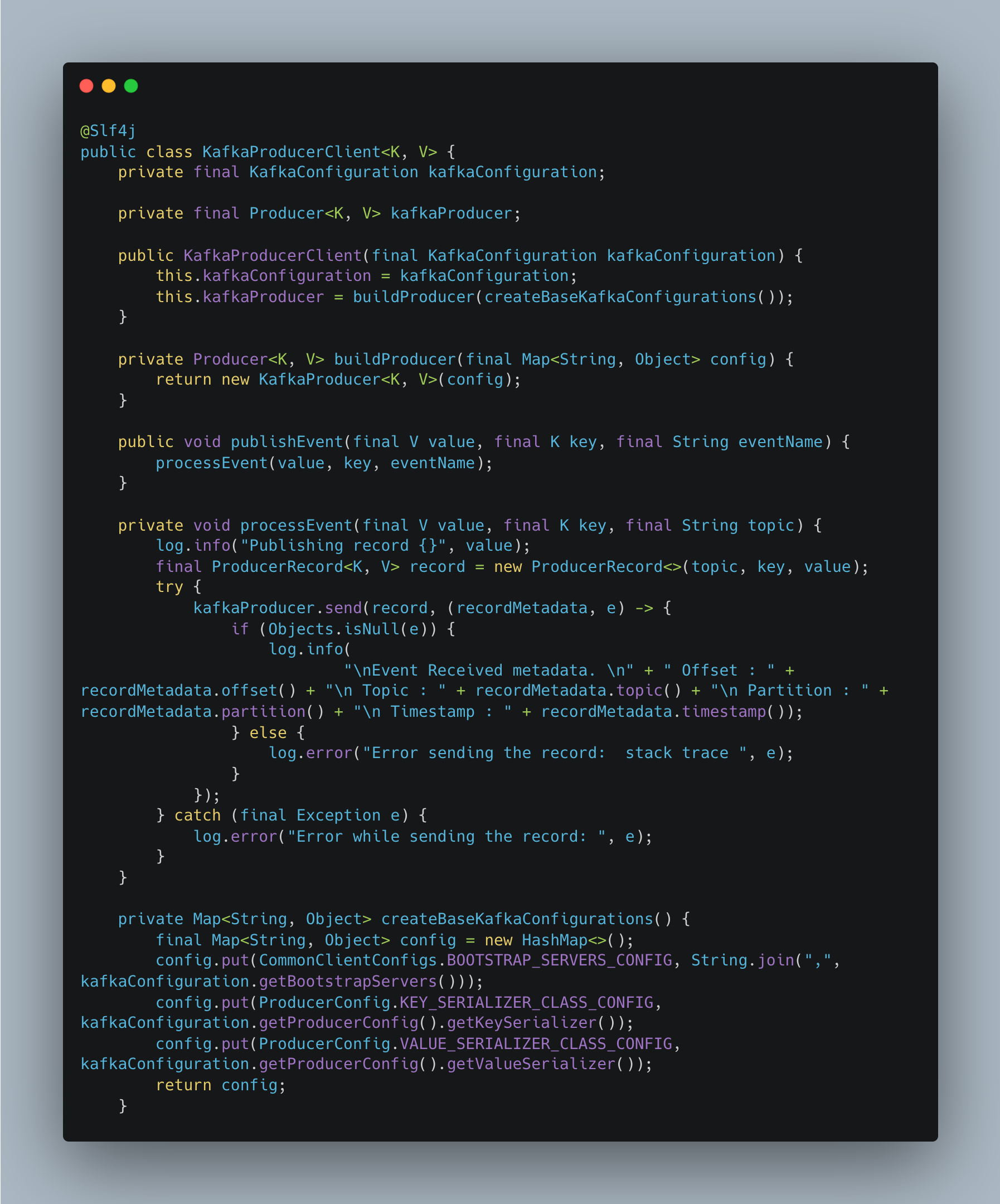
Consumer Class:
- This serves as a client for consumers. In this class, we leverage the
Runnable
interface to process records in a new thread. Within this class, thepoll()
method is utilized to consume messages from specified topics.
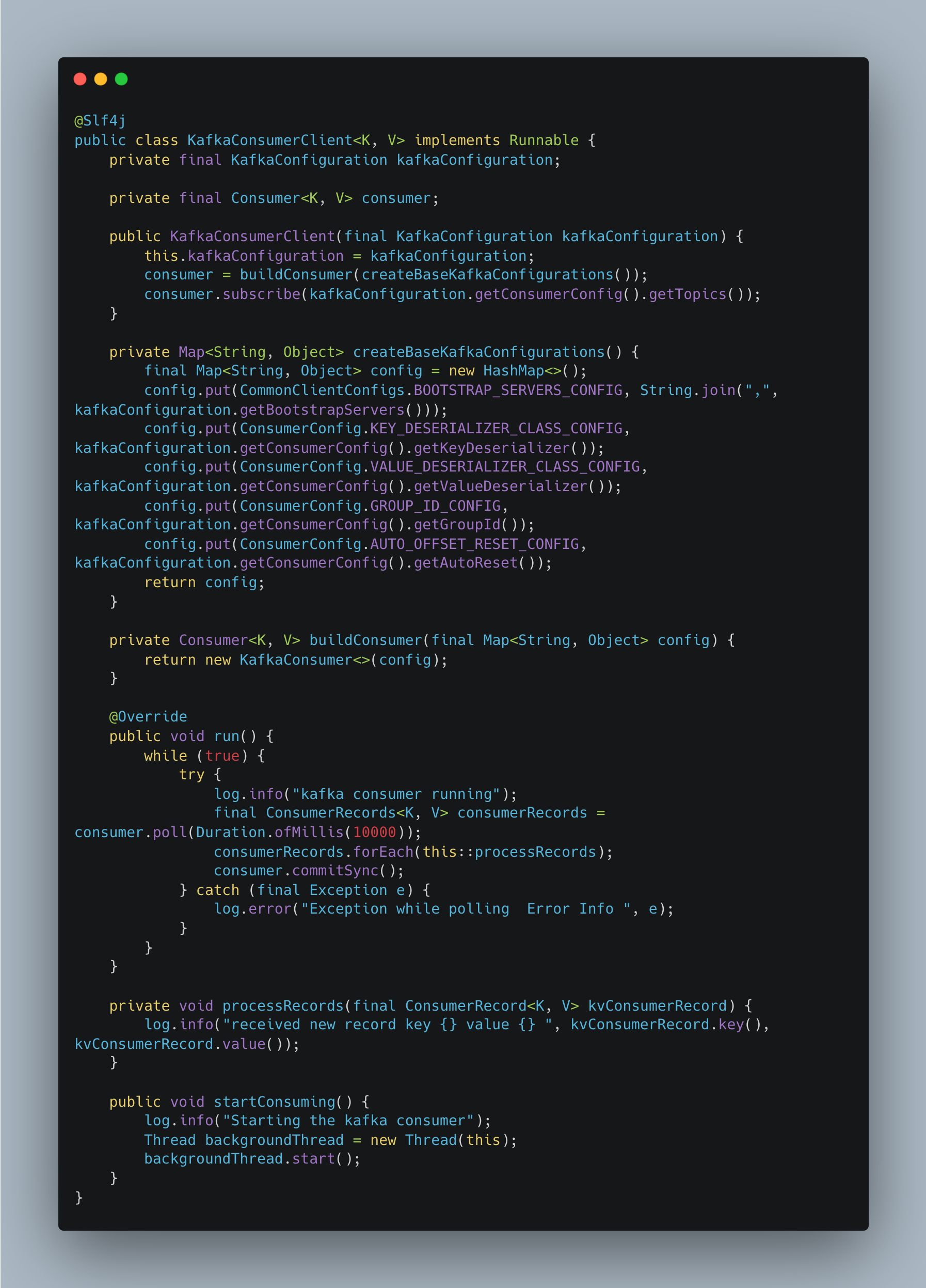
Step 5: Building SDK
With the logic implementation completed, we are now ready to package this as an SDK.
- Create a Maven Module:
- In the project's
pom.xml
, define your SDK as a Maven module. Specify dependencies, build configurations, and the main class if applicable.
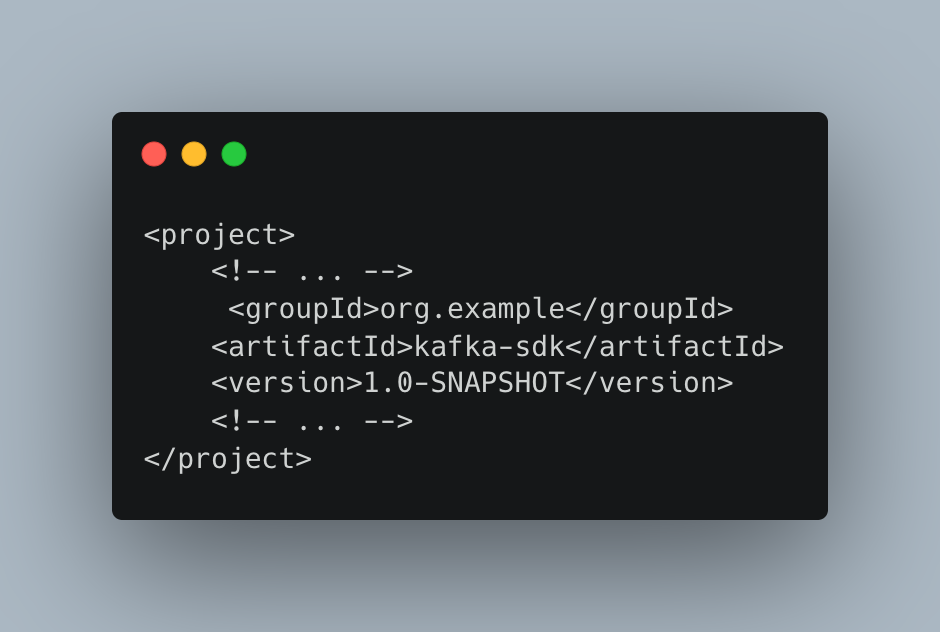
2. Build Configuration:
- Configure the build settings for your project. Ensure that the final artifact will be packaged as a JAR file.
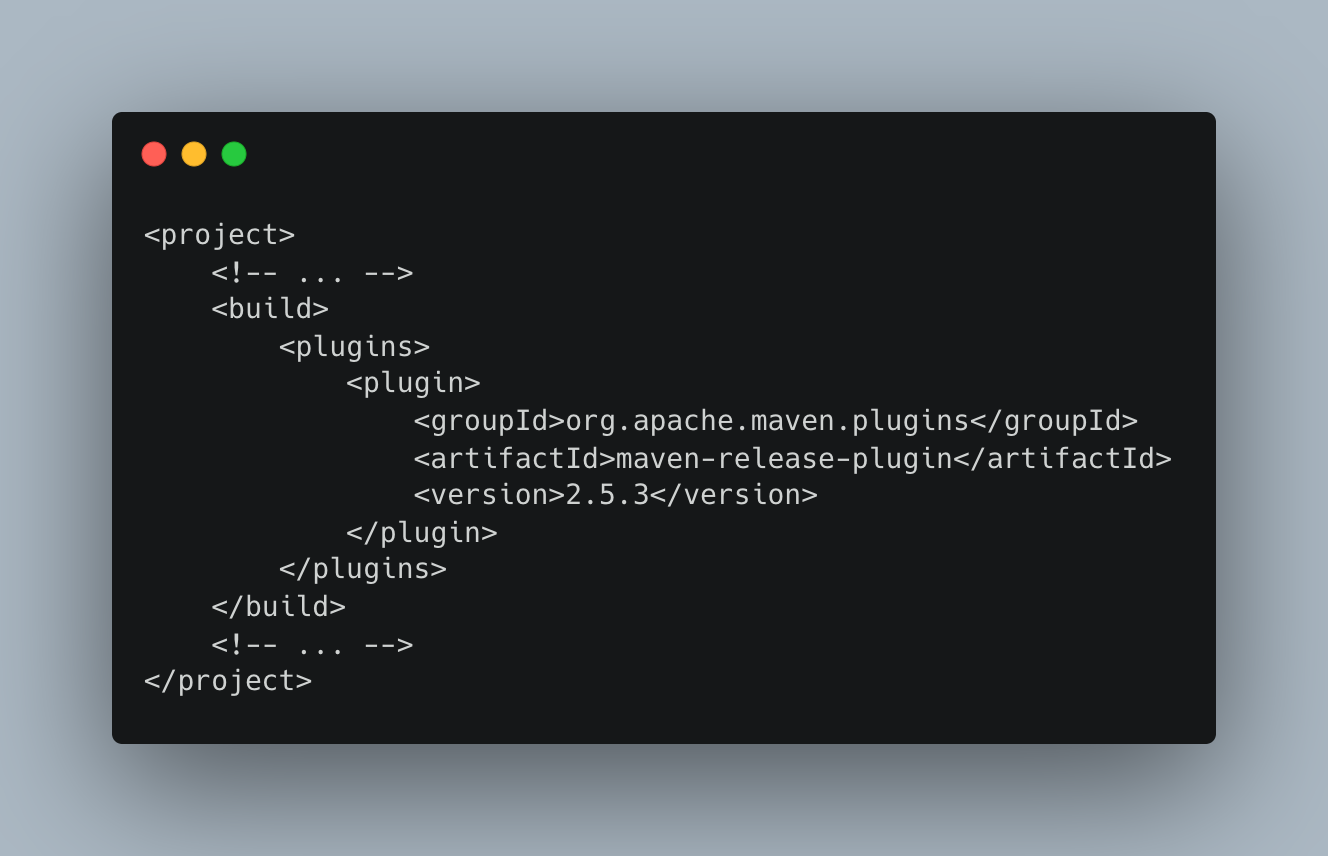
3. Build and Package:
- Use Maven to build and package your SDK. Run the following command in the project's root directory:
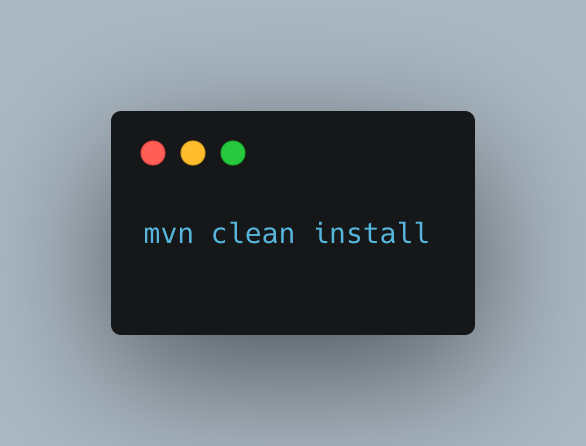
4. Distribute the JAR File:
- The resulting JAR file can be found in the
target
directory. Users can include this JAR file as a dependency in their projects.
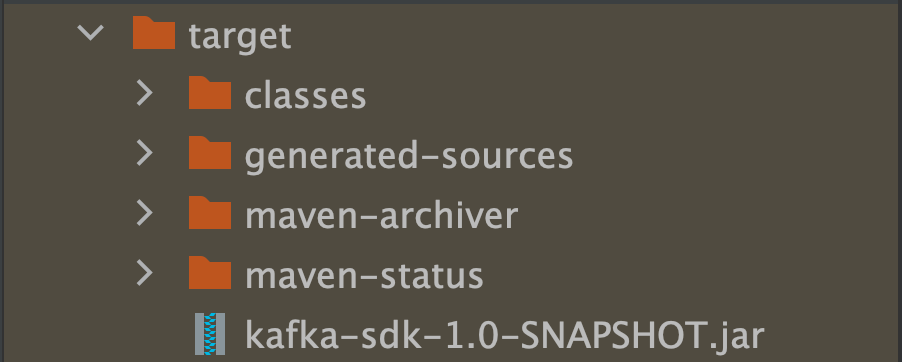
Step 6: Usage Example
Create an example class to demonstrate how to use your SDK:
- In our service , we need to add kafka-sdk dependency which we just developed.
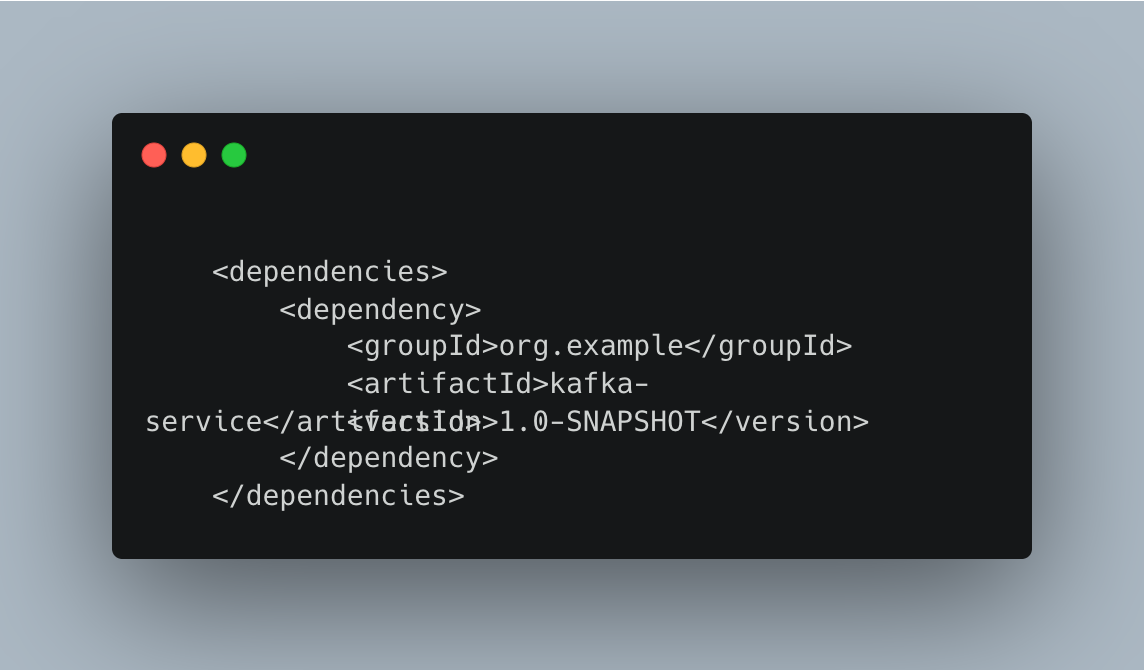
2. Following the inclusion of the necessary dependency, we can now employ the Kafka producer and consumer clients to streamline Kafka-related processes.
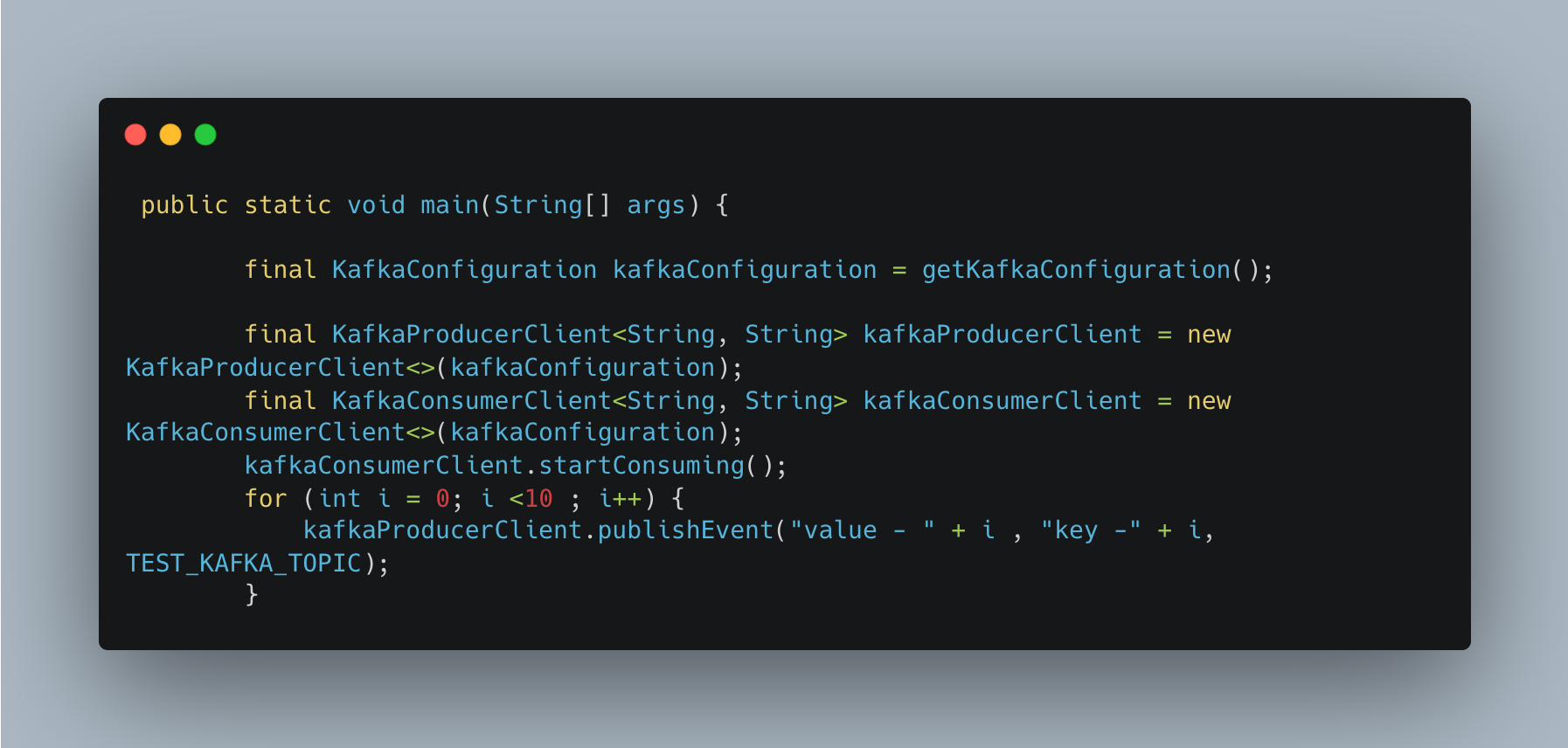
Step 7: Documentation
Create comprehensive documentation for your SDK, including setup instructions, examples, and API references.
Step 8: Testing and Validation
Thoroughly test your SDK in different scenarios to ensure reliability and functionality.
Step 9: Continuous Improvement
Regularly update your SDK based on user feedback, evolving requirements, and changes in dependencies.
Congratulations! You've now created a simple Kafka SDK for event publishing and consumption. Customize and expand the SDK according to your project's needs.
Challenges
While Software Development Kits (SDKs) offer a myriad of advantages, they are not without their challenges. Here are some common challenges associated with the use of SDKs:
Compatibility Issues:
- Version Mismatch: Developers may encounter compatibility issues when using different versions of SDKs or integrating SDKs with other tools and libraries. Ensuring that all components work seamlessly together can be a significant challenge.
Learning Curve:
- Complexity: Some SDKs, especially those designed for advanced functionalities, can have a steep learning curve. Developers may need time to familiarize themselves with the intricacies of the SDK, potentially slowing down the onboarding process.
Size and Performance:
- Bloat: SDKs can contribute to the size of an application, especially if they come with a wide range of features. This can affect the performance of the application, leading to longer load times and increased resource consumption.
Security Concerns:
- Vulnerabilities: SDKs may have security vulnerabilities that, if not promptly addressed, could pose risks to the overall security of an application. Developers need to stay vigilant for updates and patches provided by the SDK maintainers.
Dependence on Third-party Providers:
- Reliability: Relying on third-party providers for SDK updates and maintenance introduces a level of dependency. If a provider discontinues support or fails to address critical issues promptly, it could impact the stability of the application.
- Third-Party Dependency Version Conflicts: Managing version conflicts with third-party dependencies can pose a challenge, especially when integrating SDKs into projects with varying dependency requirements.
Limited Platform Support:
- Platform Dependency: Some SDKs may be designed for specific platforms or environments, limiting their cross-compatibility. This can be a challenge when developing applications for diverse platforms and devices.
- Difficulty in Incorporating Specific Use Case Features: Adapting the SDK to cater to specific use cases, especially those not applicable to a broader audience, can be challenging. Striking a balance between flexibility and simplicity becomes crucial.
Ongoing Maintenance:
- Updates and Legacy Support: Managing updates and ensuring backward compatibility with older versions of an SDK can be a time-consuming process. Legacy support becomes crucial for applications that continue to use older SDK versions.
Life Cycle Management:
- Complex Life Cycle Management: While introducing new features may be straightforward, managing the life cycle of an SDK becomes complex. Upgrading and removing features require careful consideration, often involving deprecation strategies and compatibility flags to ensure a smooth transition for existing users.
Understanding and addressing these challenges are vital for developers and teams integrating SDKs into their projects. Thorough research, careful consideration of requirements, and proactive maintenance practices can help mitigate these challenges and ensure a more seamless development experience.
Do's and Don'ts While Developing SDKs
Do's:
- Provide comprehensive documentation that explains the purpose, functionalities, and usage of the SDK. Include clear code examples, use cases, and troubleshooting tips to facilitate smooth integration by developers.
- Implement clear versioning for the SDK. Clearly communicate any changes, updates, or deprecations in subsequent versions. This helps developers understand the evolution of the SDK and manage transitions smoothly.
- Design the SDK in a modular and extensible manner. Break down functionalities into smaller, independent modules to allow developers to use only what they need and minimize the impact of updates on unrelated components.
- Implement robust error handling mechanisms. Clearly define error messages, codes, and recovery options. This ensures that developers can easily identify and troubleshoot issues during integration.
- Regularly test the SDK for compatibility with various platforms, frameworks, and environments. Ensure that the SDK seamlessly integrates with popular development tools to accommodate a broad user base.
- Prioritize security during development. Regularly audit the code for vulnerabilities, provide secure communication options, and offer guidelines for secure usage. Promptly address any reported security issues with timely updates.
- Optimize the SDK for performance. Minimize resource consumption, reduce latency, and employ efficient algorithms. A well-optimized SDK contributes to a positive user experience for applications using it.
- Implement granular permissions and access controls. Allow developers to customize the level of access the SDK has to sensitive data or functionalities, ensuring a balance between flexibility and security.
- Implement a robust continuous integration and deployment (CI/CD) pipeline. Automated testing and deployment processes help catch issues early, streamline updates, and maintain a reliable SDK.
- Incorporate unit testing along with its corresponding coverage analysis to evaluate the effectiveness of the code testing process.
Don'ts:
- Over-rely on external services or dependencies that are beyond your control. While some dependencies are unavoidable, excessive reliance can lead to issues if the external service experiences disruptions.
- Release an SDK without thorough testing. Inadequate testing can lead to undiscovered bugs, security vulnerabilities, and compatibility issues, resulting in a poor developer experience.
- Use ambiguous or restrictive licensing terms. Clearly communicate the terms of use, distribution, and any restrictions associated with the SDK to avoid legal complications for developers.
- Disregard user feedback. Actively listen to the developer community, address reported issues promptly, and consider suggestions for improvement. Ignoring feedback may lead to dissatisfaction and abandonment of the SDK.
- Roll out updates without proper communication or without considering backward compatibility. Sudden changes can disrupt existing integrations and create frustration among developers.
- Neglect versioning strategies. Failing to clearly communicate changes between versions can confuse developers, hinder updates, and impact the stability of applications using the SDK.
- Overcomplicate the SDK implementation. Strive for simplicity in usage, minimizing the learning curve for developers. A straightforward integration process contributes to the SDK's adoption.
- Develop an SDK without considering the diversity of platforms, devices, and environments where it may be used. Ensure compatibility across a range of scenarios to maximize the SDK's utility.
- Neglect security measures for communication. Use secure protocols, encrypt sensitive data, and provide guidelines for developers to follow secure practices when using the SDK.
- Abandon the SDK without regular maintenance. Keep the SDK up-to-date with the latest technologies, address bugs promptly, and provide ongoing support to maintain its relevance and usefulness.
By adhering to these do's and don'ts, SDK developers can create a robust, user-friendly, and reliable toolkit that adds value to the broader developer community.
Conclusion
In the fast-paced realm of software development, tapping into the capabilities of SDKs can be the determining factor in a project's success. Java SDKs, in particular, provide a robust toolkit, empowering developers to create scalable, efficient, and feature-rich applications. Through the exploration of real-world success stories, our aim has been to inspire developers, guiding them in unlocking the full potential of Java SDKs and raising the bar for software solutions.
In addition to our exploration of existing Java SDKs, we've taken a hands-on approach by building our own custom SDK tailored for real-life use cases. This practical endeavor not only reinforces the versatility of SDKs but also underscores their applicability in addressing specific development needs. As we conclude, we invite you to stay tuned for more in-depth explorations of specific Java SDKs and their practical applications in our upcoming posts.
References
- https://aws.amazon.com/what-is/sdk/
- https://www.adjust.com/glossary/sdk/
- https://en.wikipedia.org/wiki/Software_development_kit
Additional info:-
Here are some of the blogs our team has published, each offering in-depth insights into how we streamline our development process at halodoc . Please feel free to explore these blogs to gain deeper insights:
- https://blogs.halodoc.io/what-is-event-driven-architecture-how-halodoc-is-building-event-centric-systems-using-kafka/
- https://blogs.halodoc.io/db-transactions-in-go/
- https://blogs.halodoc.io/kafka-integration-testing-automation/
Join us
Scalability, reliability and maintainability are the three pillars that govern what we build at Halodoc Tech. We are actively looking for engineers at all levels and if solving hard problems with challenging requirements is your forte, please reach out to us with your resumé at careers.india@halodoc.com.
About Halodoc
Halodoc is the number 1 all around Healthcare application in Indonesia. Our mission is to simplify and bring quality healthcare across Indonesia, from Sabang to Merauke. We connect 20,000+ doctors with patients in need through our Tele-consultation service. We partner with 3500+ pharmacies in 100+ cities to bring medicine to your doorstep. We've also partnered with Indonesia's largest lab provider to provide lab home services, and to top it off we have recently launched a premium appointment service that partners with 500+ hospitals that allow patients to book a doctor appointment inside our application. We are extremely fortunate to be trusted by our investors, such as the Bill & Melinda Gates Foundation, Singtel, UOB Ventures, Allianz, GoJek, Astra, Temasek, and many more. We recently closed our Series D round and in total have raised around USD$100+ million for our mission. Our team works tirelessly to make sure that we create the best healthcare solution personalised for all of our patient's needs, and are continuously on a path to simplify healthcare for Indonesia.