Upgrade to ESLint 9: Boost Code Quality in Your Angular Project
As developers, we’re always striving for efficiency. Inefficiencies in code reviews, missed issues in automation, and inconsistencies in development workflows can contribute to technical debt and reduce productivity. However, as projects grow, enforcing best practices across a team becomes increasingly difficult. Gaps in code reviews, overlooked issues in automation, and inconsistencies in development workflows can lead to technical debt and slow down productivity.
Managing linting rules effectively is also a challenge. Without the right tooling, ensuring that code stays clean, readable, and aligned with project standards can become tedious. To overcome these challenges, we upgraded to ESLint 9 and refined our Jenkins + SonarQube integration. This update has helped us enhance rule enforcement, improve maintainability, and make our development workflow more efficient. In this blog, I’ll walk you through our experience and why upgrading to ESLint 9 might be a great choice for your project.
Our First ESLint Implementation
Before we upgraded to ESLint 9, we were using an older setup where ESLint, along with Prettier and tools like Husky, helped enforce coding standards and automatic formatting at the commit level. In a previous blog post, I shared how we configured this setup to ensure team-wide consistency and eliminate manual formatting reviews. If you’re curious about how we configured ESLint with Prettier, please check out the details in that post.
While this setup worked well for us, we soon realized that newer versions of ESLint offered better improvements that could further enhance our workflow. That’s why we decided to upgrade to ESLint 9
Why you should upgrade to ESLint 9?
As our codebase evolved and Angular 18 introduced new features, upgrading to ESLint 9 wasn’t just about keeping up with the latest trends—it was about making our development process smoother and easier to maintain. The new version comes with some really useful upgrades that have a direct impact on our Angular 18 projects. From smarter linting rules to better compatibility with our tools. Here’s what really caught our attention
ESLint 9 Provides Faster Linting
As our Angular project grew, we often faced slow linting times, especially in CI/CD pipelines and during local development. ESLint 9 introduces several optimizations that significantly reduce the time required to analyze code:
- Parallel Processing Enhancements: The new version improves how it distributes workload across CPU cores, making linting more efficient, particularly in larger projects.
- Smarter File Caching: ESLint 9 refines its caching mechanism, ensuring that unchanged files are skipped in subsequent linting runs, leading to faster feedback loops.
- Optimized Rule Execution: Some frequently used rules have been rewritten for better performance, reducing the overall linting time.
- Improved TypeScript Handling: The way ESLint 9 processes TypeScript has been optimized, allowing it to analyze modern syntax more efficiently without unnecessary overhead.
Built-in Support for Flat Config
Previously, ESLint relied on .eslintrc
files, which followed a hierarchical, JSON/YAML-based configuration system. While this worked well for most projects, it had limitations:
- Complex Merging Rules: Configurations were deeply nested, and resolving overrides across multiple
.eslintrc
files could be unpredictable. - Performance Overhead: Parsing multiple configuration files in large monorepos or multi-package repositories slowed down linting.
- Limited Dynamic Configuration: The old system didn’t support JavaScript-based configuration natively, making it harder to set up dynamic rule enforcement.
Benefits of Flat Config
Flat Config, introduced in ESLint 9, addresses these issues by simplifying how configurations are structured and applied. Here’s why we found it beneficial in our Angular project:
- Single Configuration File: Instead of dealing with multiple
.eslintrc
files, we now define all our ESLint rules in a singleeslint.config.js
file. - JavaScript-Based Setup: Since it’s a standard JavaScript module, we can use functions, variables, and logic to create dynamic rule configurations based on the environment (e.g., different rules for test files vs. production code).
- Faster Processing: Flat Config eliminates the need for deep merging, reducing linting overhead and improving performance, especially in larger projects.
- Better Extensibility: It simplifies extending third-party configurations (e.g., TypeScript, Prettier, Angular) without the confusion of nested extends arrays.
Flat Config Example:
Here’s how we set up Flat Config in our eslint.config.js
file:
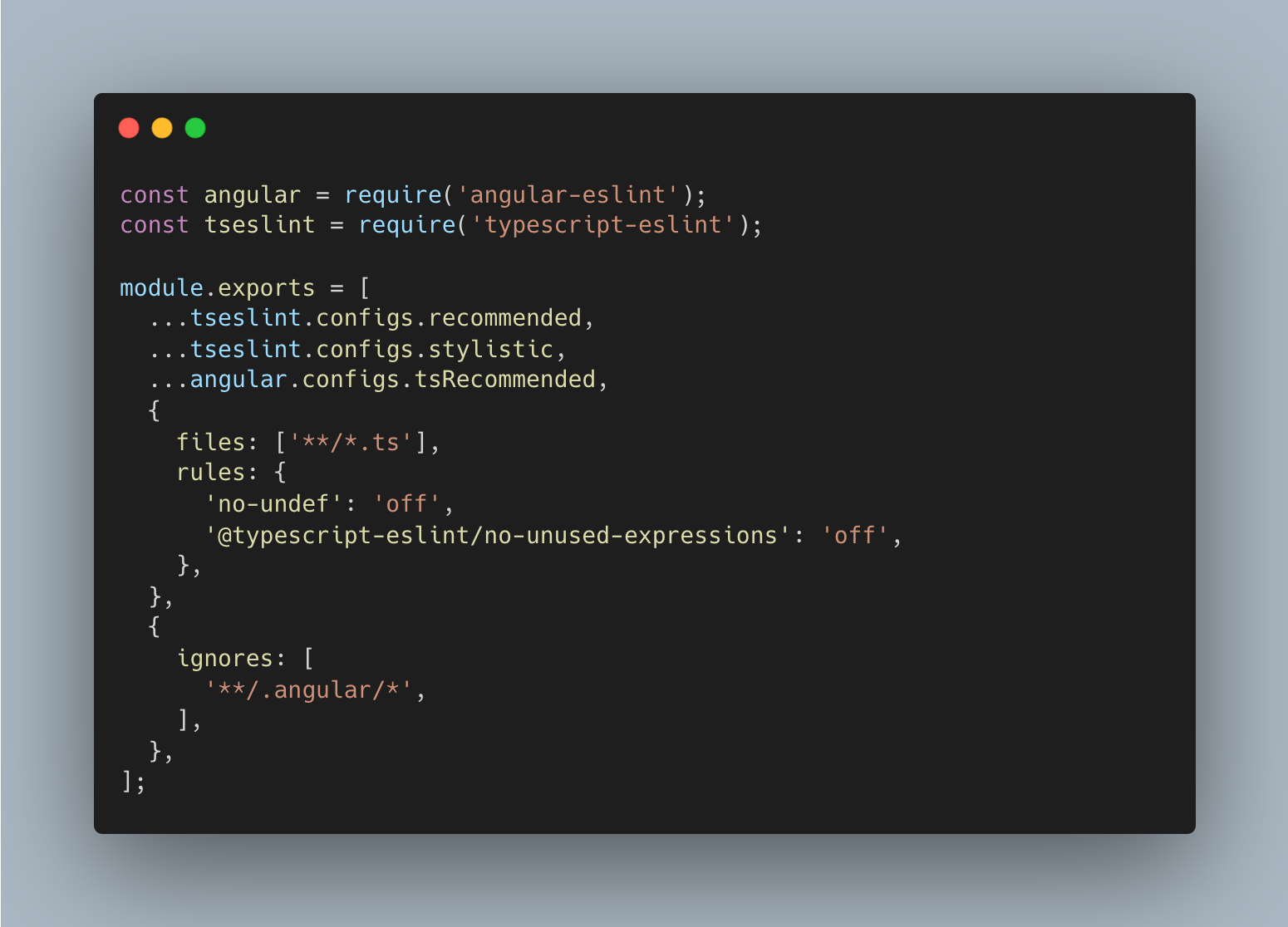
More Flexible and Granular Configuration
ESLint 9 introduces Flat Config, which enhances flexibility when defining overrides for specific files or rules. This allows us to enforce different linting standards across test files, production code, and other environments with better precision.
Why This Matters
Previously, configuring overrides in .eslintrc.js
could become complex due to deep merging and hierarchical configurations. With Flat Config, ESLint 9 simplifies this by using a single JavaScript-based configuration (eslint.config.js
), allowing more dynamic rule application.
Example: Setting Different Rules for Test and Production Files
Let’s say we want to allow console logs in test files but disallow them in production. With ESLint 9, we can configure this cleanly:
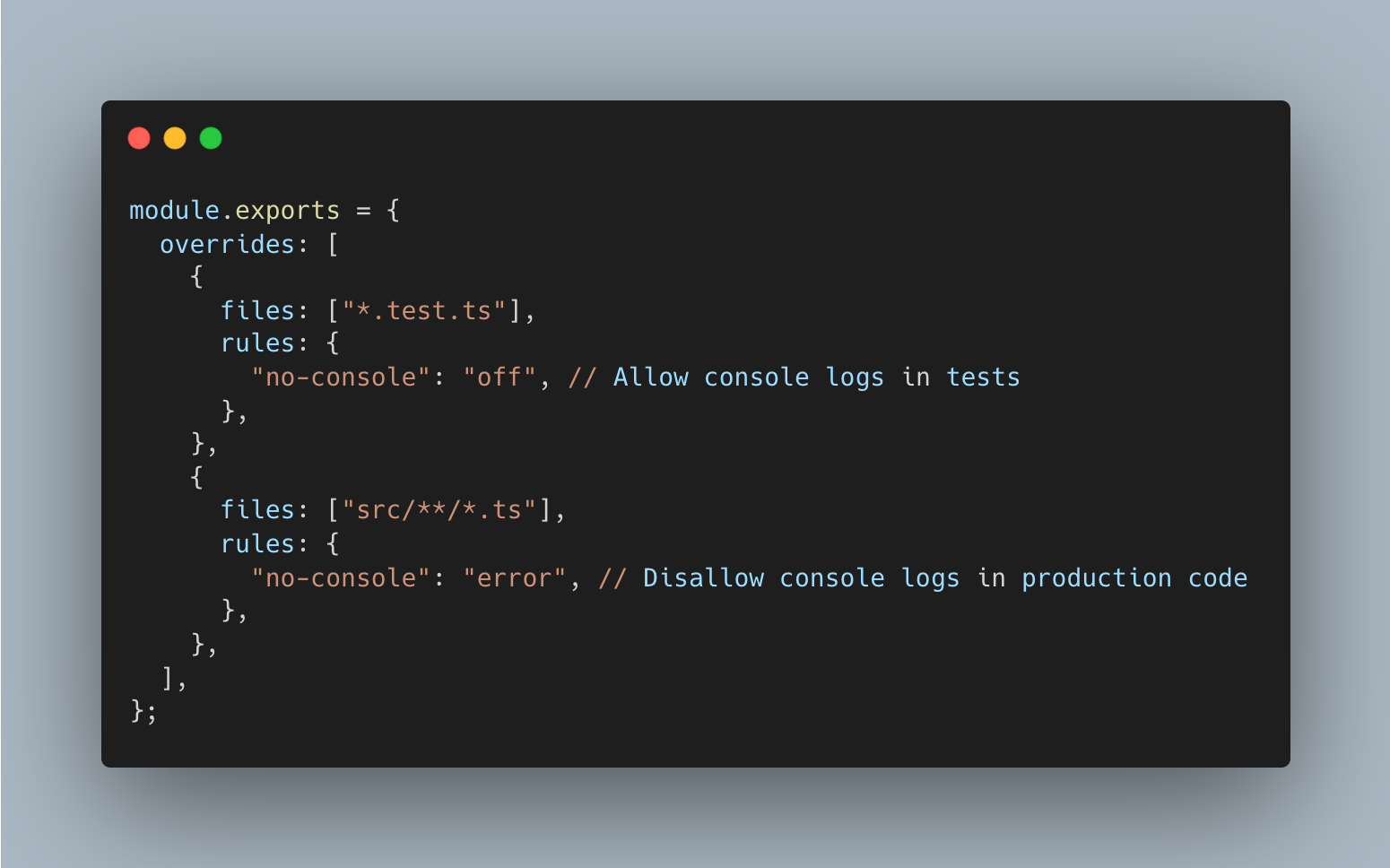
Smarter Rules and Stronger TypeScript Support
One of the standout features of ESLint 9 is its improved TypeScript support. Given Angular 18’s heavy reliance on TypeScript, This upgrade really helped us. The new version understands modern syntax and patterns, reducing false positives and ensuring our code aligns with best practices. For example, older versions of ESLint often flagged Angular decorators incorrectly, but ESLint 9 handles them seamlessly.
Before ESLint 9 (False Positive Warning)
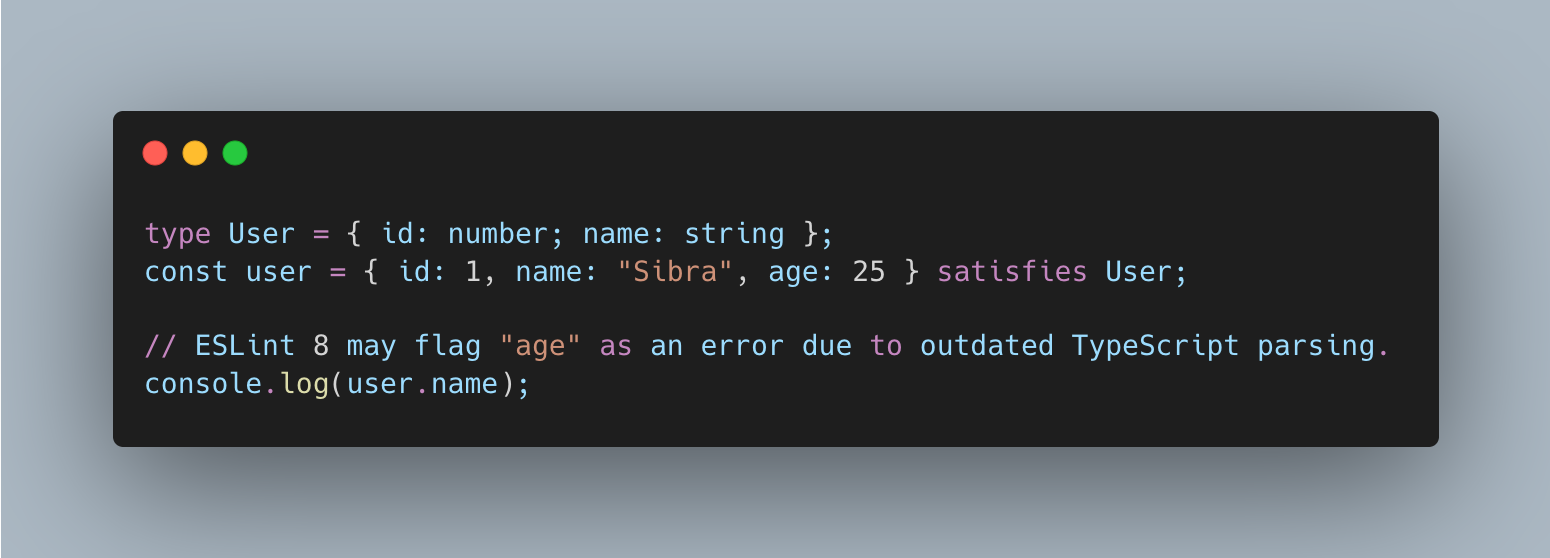
After ESLint 9 (Proper Parsing)
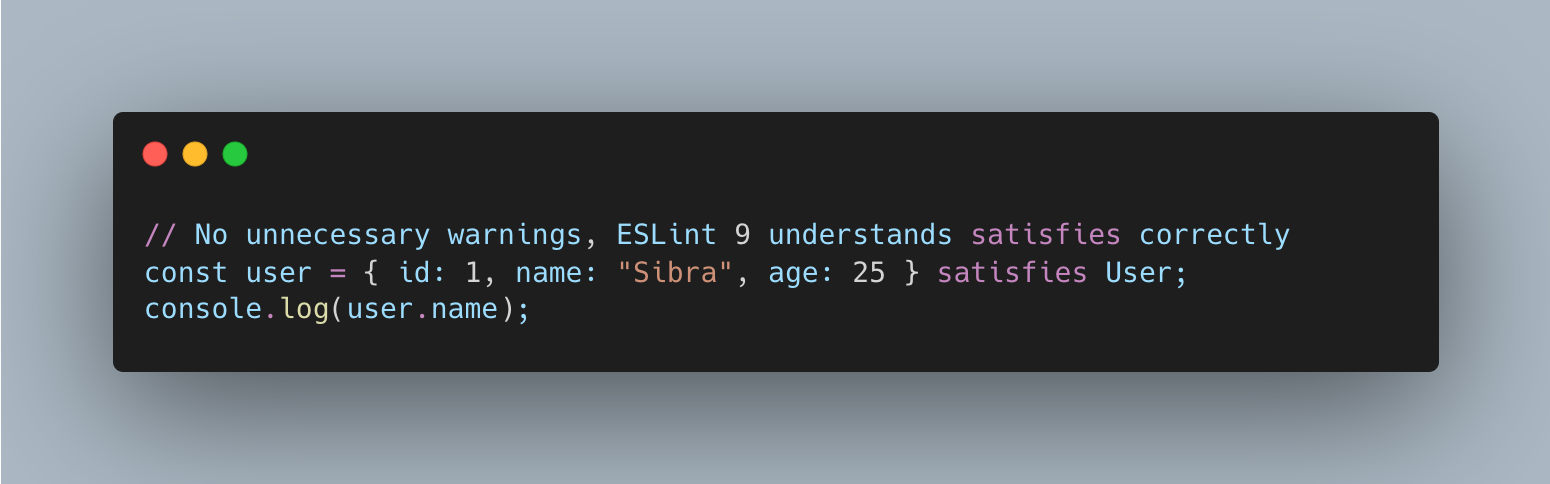
We’ve not only minimized unnecessary warnings but also reduced ignored lint rules, resulting in a more maintainable codebase. Developers can now confidently use advanced TypeScript features without worrying about outdated parsing issues from previous versions.
Smoother Integration with Sonar and CI/CD Pipelines
We’ve got Jenkins integrated with Sonar to catch ESLint errors at the pipeline level, and upgrading to ESLint 9 made this process even smoother. The new version provides more detailed error outputs, which makes debugging issues in CI/CD way easier—before they ever hit production.
To enhance this workflow, we added this script in package.json
to generate an ESLint report
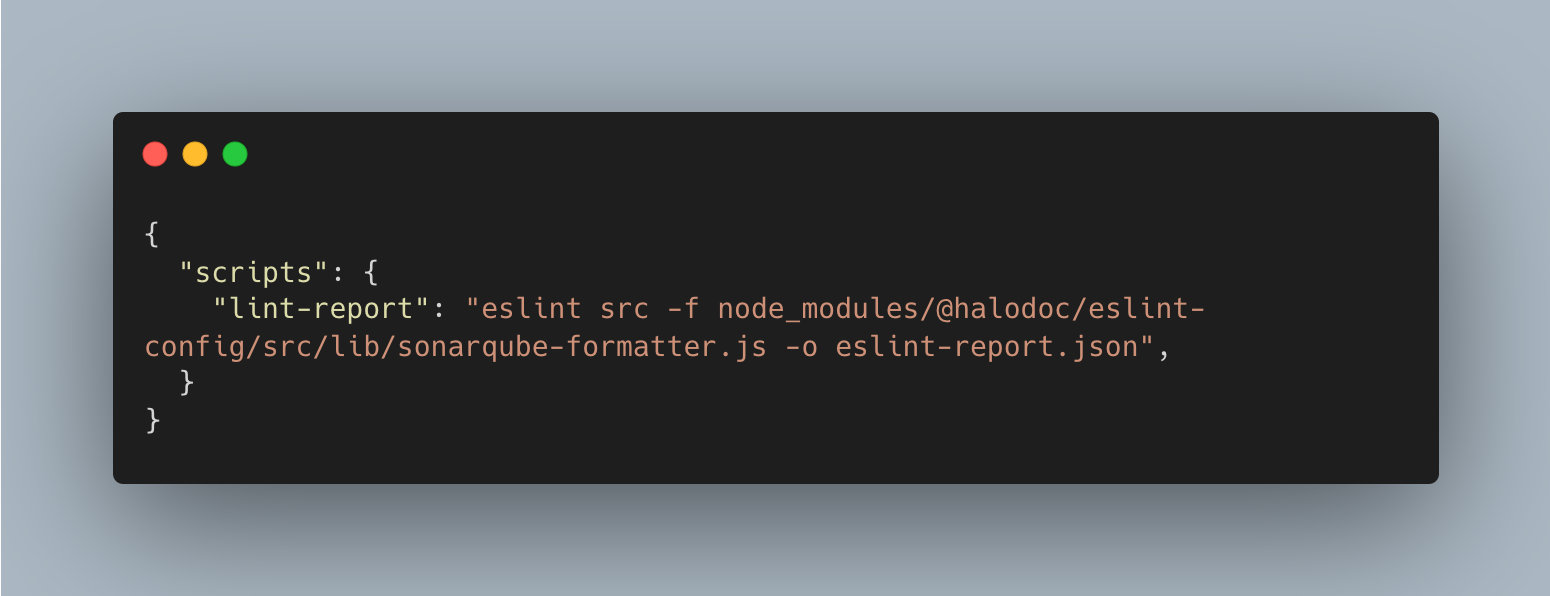
Next, we implemented a SonarQube formatter to process the ESLint report and ensure seamless mapping within SonarQube. This formatter translates ESLint findings into a format that SonarQube can accurately interpret, allowing us to track and manage linting issues directly within our SonarQube dashboard. This integration helps maintain code quality by consolidating all ESLint errors in one place.
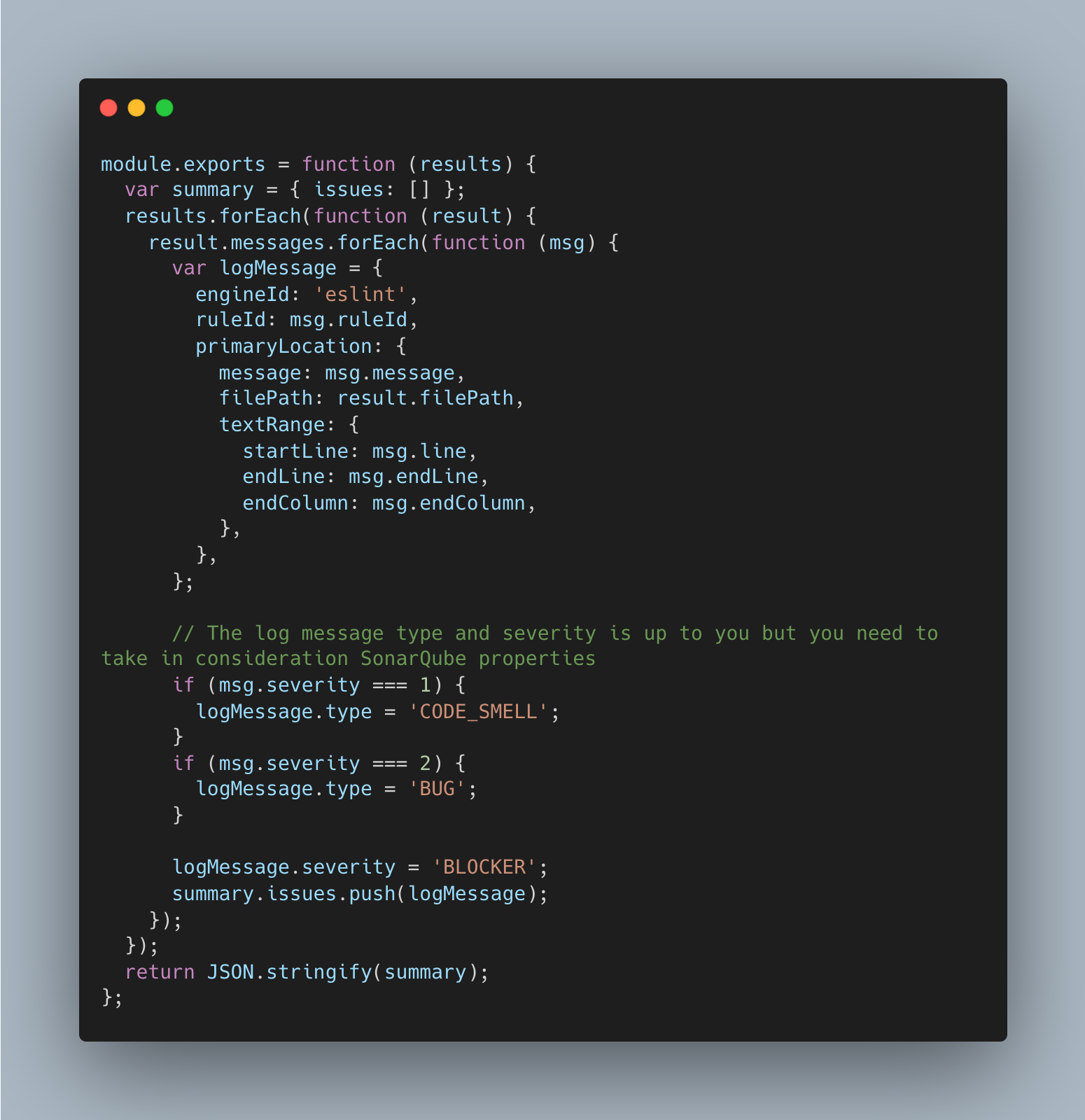
After exporting the ESLint report, SonarQube automatically picks it up and analyzes the results. If any ESLint errors are detected, SonarQube flags them as issues, ensuring they are visible in the quality reports. These flagged errors are then fed into our CI/CD pipeline, where Jenkins validates the results. If any critical ESLint errors are present, Jenkins marks the build as failed, preventing non-compliant code from being merged or deployed.

With this error message in SonarQube, developers can easily check the error and fix it directly because they can see more detailed information here.
Custom Rules Made Easier
One of the coolest things about ESLint 9 is how flexible it is when it comes to custom rules. As our codebase grows, we’ve got specific coding standards that need to be enforced. The new version makes it simpler to extend configurations, tweak rules, and keep everything consistent across the board.
A great example of this in our workflow is our custom ESLint rule that prevents developers from adding istanbul ignore
comments. This ensures that all code is properly tested, reinforcing our commitment to maintaining high test coverage and overall code quality.
First, we implemented a custom ESLint rule called "No Istanbul Ignore"
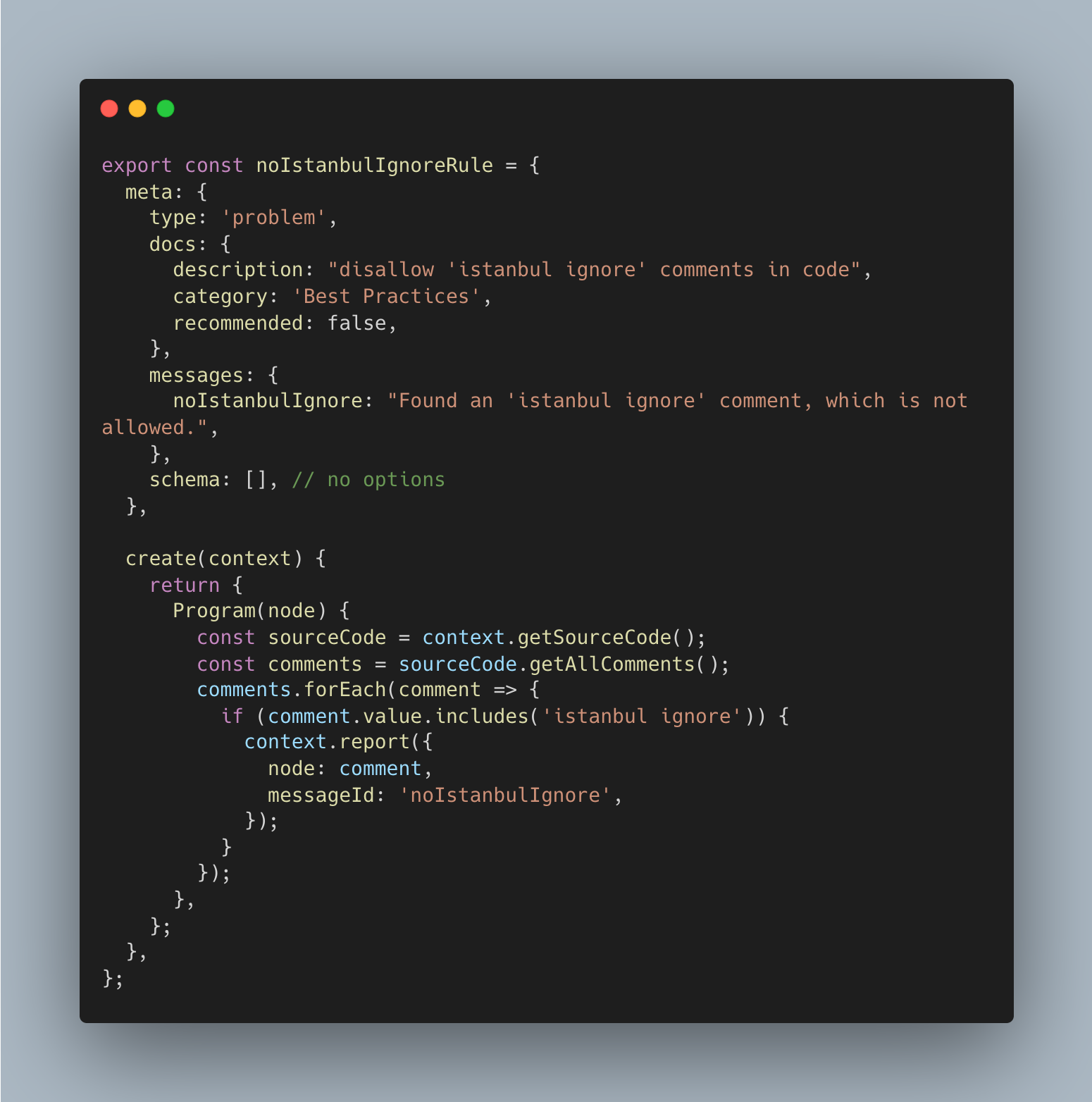
Next, we integrated the "No Istanbul Ignore" custom rule into our ESLint configuration. By adding it to our ESLint setup
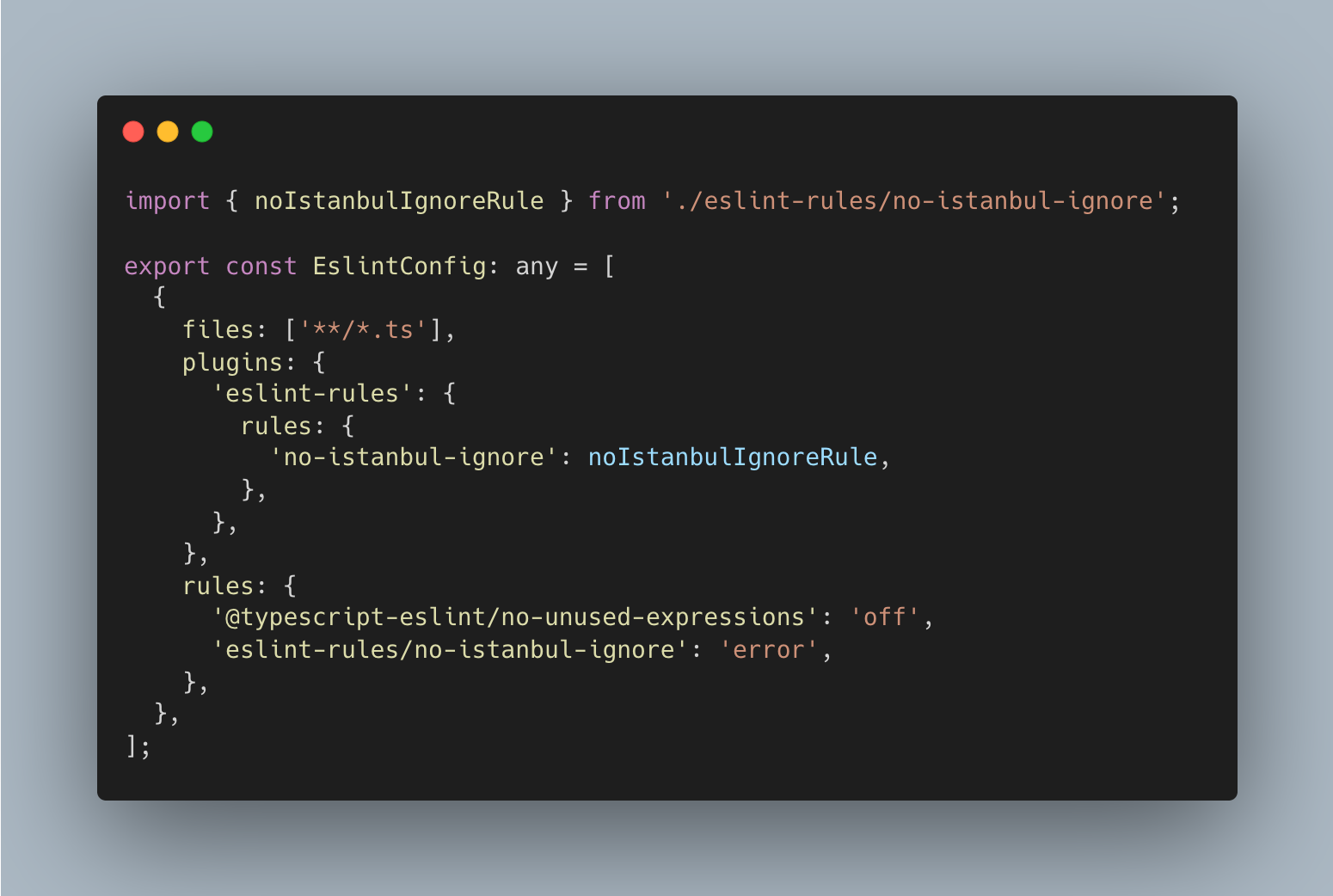
With the rule integrated, it runs in pre-commit hooks to prevent ignored coverage before commits and in the CI/CD pipeline to block untested code from being merged or deployed.
This is just one example of our custom ESLint rule in ESLint 9. You can create additional rules to enforce other project-specific coding standards.
Lessons We Learned Along the Way
- Upgrade Dependencies Carefully: Some ESLint plugins and rules had breaking changes, so we had to update our dependencies step by step to avoid issues.
- Run Tests After Migration: After the migration, we ran our test suite to make sure nothing broke unexpectedly.
- Share with the Team: A new ESLint version means new rules and behaviors. We held a quick team session to go over the changes and explain how they’d impact our workflow.
Conclusions
Migrating to ESLint 9 has improved our Angular 18 development workflow. With smarter TypeScript support, seamless SonarQube and CI/CD integration, and easier custom rule creation, we’ve strengthened code quality and maintainability. The transition also reinforced best practices, ensuring consistent linting, better test coverage enforcement, and reduced false positives. While there were a few challenges—like updating dependencies and adjusting configurations—the benefits far outweighed the effort. If you're using ESLint, upgrading to version 9 is worth considering, regardless of your framework.
References
- Automatically formatting and cleaning code on commit
- Migrate to ESLint 9
- ESLint Version Upgrade Guide (v8 to v9) for Angular Projects
Join us
Scalability, reliability, and maintainability are the three pillars that govern what we build at Halodoc Tech. We are actively looking for engineers at all levels and if solving hard problems with challenging requirements is your forte, please reach out to us with your resumé at careers.india@halodoc.com
About Halodoc
Halodoc is the number 1 Healthcare application in Indonesia. Our mission is to simplify and bring quality healthcare across Indonesia, from Sabang to Merauke. We connect 20,000+ doctors with patients in need through our Tele-consultation service. We partner with 3500+ pharmacies in 100+ cities to bring medicine to your doorstep. We've also partnered with Indonesia's largest lab provider to provide lab home services, and to top it off we have recently launched a premium appointment service that partners with 500+ hospitals that allow patients to book a doctor appointment inside our application. We are extremely fortunate to be trusted by our investors, such as the Bill & Melinda Gates Foundation, Singtel, UOB Ventures, Allianz, GoJek, Astra, Temasek, and many more. We recently closed our Series D round and in total have raised around USD$100+ million for our mission. Our team works tirelessly to make sure that we create the best healthcare solution personalized for all of our patient's needs, and are continuously on a path to simplify healthcare for Indonesia.